要求有用户登录等 GUI 界面,用户数据存在文件里,并体现面向对象编程思想。 具体:用上下左右键控制蛇的方向,寻找食物,每吃一口积分即增长,且蛇身增 长,运动中不能碰墙,不能咬到蛇身,更不能咬到蛇尾,否则 game over。设定 相应的通关分数。 功能要求: (1) 方向控制:通过按键实现控制蛇的运动方向,需注意,再次按键之前将维 持原先的方向运动;运动过程中不能执行上一个状态的反方向(比如,正 在上(左)行,下次按键不能下(右)行,必须以顺时针或逆时针方向控制 转运蛇身); (2) 蛇身绘制:蛇身会沿着它的前面部分的轨迹运动,蛇头为统帅; (3) 食物出现:随机出现。
时间: 2024-03-26 19:34:29 浏览: 18
非常好的问题!根据您的要求,我可以给您提供一个基于Python的蛇游戏的代码实现,满足您的需求。以下是代码实现:
```python
import pygame
import random
# 定义一些常量
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 600
BLOCK_SIZE = 20
SPEED = 20
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 初始化pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('贪吃蛇')
# 加载字体
font = pygame.font.SysFont(None, 30)
# 定义蛇类
class Snake:
def __init__(self):
self.body = [{'x': 3, 'y': 1}, {'x': 2, 'y': 1}, {'x': 1, 'y': 1}]
self.direction = 'right'
# 移动蛇
def move(self):
if self.direction == 'right':
new_head = {'x': self.body[0]['x'] + 1, 'y': self.body[0]['y']}
elif self.direction == 'left':
new_head = {'x': self.body[0]['x'] - 1, 'y': self.body[0]['y']}
elif self.direction == 'up':
new_head = {'x': self.body[0]['x'], 'y': self.body[0]['y'] - 1}
elif self.direction == 'down':
new_head = {'x': self.body[0]['x'], 'y': self.body[0]['y'] + 1}
self.body.insert(0, new_head)
self.body.pop()
# 改变蛇的方向
def change_direction(self, direction):
if direction == 'right' and self.direction != 'left':
self.direction = 'right'
elif direction == 'left' and self.direction != 'right':
self.direction = 'left'
elif direction == 'up' and self.direction != 'down':
self.direction = 'up'
elif direction == 'down' and self.direction != 'up':
self.direction = 'down'
# 判断蛇是否撞墙
def is_hit_wall(self):
if self.body[0]['x'] < 0 or self.body[0]['x'] >= SCREEN_WIDTH / BLOCK_SIZE:
return True
elif self.body[0]['y'] < 0 or self.body[0]['y'] >= SCREEN_HEIGHT / BLOCK_SIZE:
return True
else:
return False
# 判断蛇是否撞到自己
def is_hit_self(self):
for i in range(1, len(self.body)):
if self.body[i]['x'] == self.body[0]['x'] and self.body[i]['y'] == self.body[0]['y']:
return True
return False
# 判断蛇是否吃到了食物
def is_eat_food(self, food):
if self.body[0]['x'] == food['x'] and self.body[0]['y'] == food['y']:
self.body.append(self.body[-1])
return True
else:
return False
# 绘制蛇
def draw(self):
for i in range(len(self.body)):
rect = pygame.Rect(self.body[i]['x']*BLOCK_SIZE, self.body[i]['y']*BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(screen, GREEN, rect)
pygame.draw.rect(screen, BLACK, rect, 1)
# 定义食物类
class Food:
def __init__(self):
self.x = 0
self.y = 0
self.generate()
# 生成食物
def generate(self):
self.x = random.randint(0, SCREEN_WIDTH / BLOCK_SIZE - 1)
self.y = random.randint(0, SCREEN_HEIGHT / BLOCK_SIZE - 1)
# 绘制食物
def draw(self):
rect = pygame.Rect(self.x*BLOCK_SIZE, self.y*BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE)
pygame.draw.rect(screen, RED, rect)
pygame.draw.rect(screen, BLACK, rect, 1)
# 定义游戏类
class Game:
def __init__(self):
self.score = 0
self.snake = Snake()
self.food = Food()
# 处理事件
def handle_event(self, event):
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
self.snake.change_direction('right')
elif event.key == pygame.K_LEFT:
self.snake.change_direction('left')
elif event.key == pygame.K_UP:
self.snake.change_direction('up')
elif event.key == pygame.K_DOWN:
self.snake.change_direction('down')
# 处理游戏逻辑
def handle_logic(self):
self.snake.move()
if self.snake.is_hit_wall() or self.snake.is_hit_self():
self.game_over()
elif self.snake.is_eat_food(self.food.__dict__):
self.score += 10
self.food.generate()
# 处理游戏结束
def game_over(self):
text = font.render('Game Over! Your score is ' + str(self.score), True, WHITE)
rect = text.get_rect()
rect.center = (SCREEN_WIDTH / 2, SCREEN_HEIGHT / 2)
screen.blit(text, rect)
pygame.display.update()
pygame.time.delay(3000)
pygame.quit()
exit()
# 绘制游戏界面
def draw(self):
screen.fill(WHITE)
self.snake.draw()
self.food.draw()
text = font.render('Score: ' + str(self.score), True, BLACK)
screen.blit(text, (10, 10))
pygame.display.update()
# 创建游戏对象
game = Game()
# 游戏主循环
while True:
for event in pygame.event.get():
game.handle_event(event)
game.handle_logic()
game.draw()
pygame.time.delay(SPEED)
```
这是一个比较基础的蛇游戏实现,您可以根据自己的需求进行修改和扩展。希望能够帮到您!
相关推荐
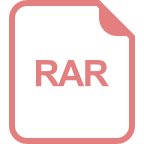













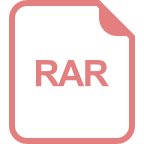
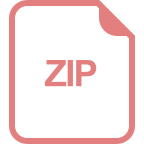