假设有如下三个基类的定义: class Base1{ int a,b,c; public: void initBase1(int a,int b,int c){ this->a=a; this->b=b; this->c=c; } int getA()const{ return a; } int getB()const{ return b; } protected: int getC()const{ return c; } }; class Base2{ int x,y,z; public: void initBase2(int x,int y,int z){ this->x=x; this->y=y; this->z=z; } int getX()const{ return x; } int getY()const{ return y; } int getZ()const{ return z; } }; class Base3{ protected: int i,j,k; void initBase3(int i,int j,int k){ this->i=i; this->j=j; this->k=k; } private: int getI()const{ return i; } int getJ()const{ return j; } int getK()const{ return k; } }; 并且有派生类的定义的第一行: class Derived:public Base2,private Base3,protected Base1 main函数已经写好了,请根据基类的定义和main函数的定义,结合输入输出,完成Derived类的定义: int main(){ int a,b,c; int x,y,z; int i,j,k; cin>>a>>b>>c; cin>>x>>y>>z; cin>>i>>j>>k; Derived d; d.initBase1(a,b,c); d.initBase2(x,y,z); d.initBase3(i,j,k); cout<<d.getA()<<" "<<d.getB()<<" "<<d.getC()<<endl; cout<<d.getX()<<" "<<d.getY()<<" "<<d.getZ()<<endl; cout<<d.getI()<<" "<<d.getJ()<<" "<<d.getK()<<endl; return 0; } 输入描述输入9个整数输出描述输出三行数据,每行三个整数提示你只需要提交Derived类的类体花括号内部的代码,Derived类定义的第一行和类体的花括号不需要提交样例输入 复制 更多样例输出
时间: 2023-12-20 08:04:02 浏览: 55
```c++
class Derived : public Base2, private Base3, protected Base1 {
public:
void initDerived(int a, int b, int c, int x, int y, int z, int i, int j, int k) {
initBase1(a, b, c);
initBase2(x, y, z);
initBase3(i, j, k);
}
using Base1::getA;
using Base1::getB;
using Base1::getC;
using Base2::getX;
using Base2::getY;
using Base2::getZ;
using Base3::getI;
using Base3::getJ;
using Base3::getK;
};
```
相关问题
假设有如下三个基类的定义: class Base1{ int a,b,c; public: void initBase1(int a,int b,int c){ this->a=a; this->b=b; this->c=c; } int getA()const{ return a; } int getB()const{ return b; } protected: int getC()const{ return c; } }; class Base2{ int x,y,z; public: void initBase2(int x,int y,int z){ this->x=x; this->y=y; this->z=z; } int getX()const{ return x; } int getY()const{ return y; } int getZ()const{ return z; } }; class Base3{ protected: int i,j,k; void initBase3(int i,int j,int k){ this->i=i; this->j=j; this->k=k; } private: int getI()const{ return i; } int getJ()const{ return j; } int getK()const{ return k; } }; 并且有派生类的定义的第一行: class Derived:public Base2,private Base3,protected Base1 main函数已经写好了,请根据基类的定义和main函数的定义,结合输入输出,完成Derived类的定义: int main(){ int a,b,c; int x,y,z; int i,j,k; cin>>a>>b>>c; cin>>x>>y>>z; cin>>i>>j>>k; Derived d; d.initBase1(a,b,c); d.initBase2(x,y,z); d.initBase3(i,j,k); cout<<d.getA()<<" "<<d.getB()<<" "<<d.getC()<<endl; cout<<d.getX()<<" "<<d.getY()<<" "<<d.getZ()<<endl; cout<<d.getI()<<" "<<d.getJ()<<" "<<d.getK()<<endl; return 0; }
根据输入输出和三个基类的定义,我们可以完成Derived类的定义:
```
class Derived : public Base2, private Base3, protected Base1 {
public:
void initDerived(int a, int b, int c, int x, int y, int z, int i, int j, int k) {
initBase1(a, b, c);
initBase2(x, y, z);
initBase3(i, j, k);
}
int getA() const { return Base1::getA(); }
int getB() const { return Base1::getB(); }
int getC() const { return Base1::getC(); }
int getX() const { return Base2::getX(); }
int getY() const { return Base2::getY(); }
int getZ() const { return Base2::getZ(); }
// Base3中的成员函数是private的,无法在Derived中访问
};
```
在main函数中,我们可以这样使用Derived类:
```
int main() {
int a, b, c;
int x, y, z;
int i, j, k;
cin >> a >> b >> c;
cin >> x >> y >> z;
cin >> i >> j >> k;
Derived d;
d.initDerived(a, b, c, x, y, z, i, j, k);
cout << d.getA() << " " << d.getB() << " " << d.getC() << endl;
cout << d.getX() << " " << d.getY() << " " << d.getZ() << endl;
// Base3中的成员函数是private的,无法在Derived中访问
return 0;
}
```
指出下面程序错误,并修改运行。 class A{ public: void setA(int); void showA(); private: int a; }; class B{ public: void setB(int); void showB(); private: int b; }; class C : public A, private B{ public: void setC(int, int, int); void showC(); private: int c; }; void A::setA(int x) { a=x; } void B::setB(int x) { b=x; } void C::setC(int x, int y, int z) { //派生类成员直接访问基类的 //公有成员 setA(x); setB(y); c=z; } void A::showA() { cout<<a<<endl; } void B::showB() { cout<<b<<endl; } void C::showC() { A::showA();B::showB();cout<<c<<endl;} int main() { C obj; obj.setA(5); obj.showA(); obj.setC(6,7,9); obj.showC(); obj.setB(6); obj.showB(); return 0; }
程序的问题在于在main函数中直接调用了C类的私有继承自B类的成员函数setB()和showB(),应该改为在C类中增加公有成员函数来访问B类的私有成员函数。以下是修改后的程序:
```
#include <iostream>
using namespace std;
class A{
public:
void setA(int);
void showA();
private:
int a;
};
class B{
public:
void setB(int);
void showB();
private:
int b;
};
class C : public A, private B{
public:
void setC(int, int, int);
void showC();
void setB_public(int x) { setB(x); }
void showB_public() { showB(); }
private:
int c;
};
void A::setA(int x) { a = x; }
void B::setB(int x) { b = x; }
void C::setC(int x, int y, int z) {
setA(x);
setB(y);
c = z;
}
void A::showA() { cout << a << endl; }
void B::showB() { cout << b << endl; }
void C::showC() { A::showA(); B::showB(); cout << c << endl; }
int main() {
C obj;
obj.setA(5);
obj.showA();
obj.setC(6, 7, 9);
obj.showC();
obj.setB_public(6);
obj.showB_public();
return 0;
}
```
在C类中增加了公有成员函数setB_public()和showB_public()来访问B类的私有成员函数,然后在main函数中通过这两个函数来调用B类的成员函数。
阅读全文
相关推荐
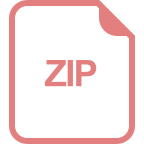
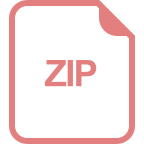














