设计一个大学的某成绩管理系统中的人员类,学校中有学生、教师、职员,每种人员都有自己的特性,他们之间又有相同的地方。利用继承机制定义这个系统中的各个类及类上必需的操作。(基类数据成员至少3个、成员函数至少一个,根据自己的理解声明即可,无需定义实现类中的成员函数)
时间: 2024-12-11 13:39:05 浏览: 4
在设计一个大学成绩管理系统中的人员类时,我们可以创建一个基类`Person`,然后分别派生出`Student`、`Teacher`和`Employee`三个子类。这些类都有一些共同的数据成员(如姓名、ID等)以及可能相同的操作,比如显示基本信息。以下是一个简化的示例:
```cpp
// 基类 Person
class Person {
public:
// 数据成员
std::string name;
int id;
double salary; // 对于职员来说
// 成员函数 - 显示基本信息 (共有的)
virtual void displayInfo() const {
std::cout << "Name: " << name << ", ID: " << id << ", ";
if (dynamic_cast<Employee*>(this)) {
std::cout << "Salary: " << salary << std::endl;
} else {
std::cout << "No salary for this type." << std::endl;
}
}
protected:
// 构造函数,只设置基本属性
Person(const std::string& n, int i) : name(n), id(i) {}
};
// 子类 Student 和 Teacher 继承自 Person
class Student : public Person {
public:
// 可能特有的数据成员和函数 (如学号、课程列表)
int studentId;
std::vector<std::string> courses;
// 如果需要,可以重写displayInfo()以添加学生信息
// override关键字确保正确覆盖父类版本
void displayInfo() const override {
Person::displayInfo();
std::cout << "Student ID: " << studentId << std::endl;
}
};
class Teacher : public Person {
public:
// 教师可能特有的数据成员 (如教龄、科目)
int teachingYears;
std::string subject;
// 同样,如果需要,可以重写displayInfo()以添加教师信息
void displayInfo() const override {
Person::displayInfo();
std::cout << "Teaching Years: " << teachingYears << ", Subject: " << subject << std::endl;
}
};
// 子类 Employee 继承自 Person 并添加额外功能
class Employee : public Person {
public:
// 数据成员 - 公司名称、职位等
std::string company;
std::string position;
// 定义薪资相关的成员函数
void setSalary(double s) { salary = s; }
// displayInfo() 的实现
void displayInfo() const override {
Person::displayInfo();
std::cout << "Company: " << company << ", Position: " << position << ", Salary: " << salary << std::endl;
}
};
```
阅读全文
相关推荐
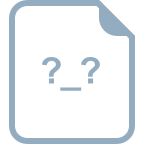
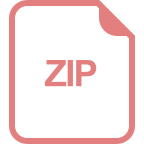
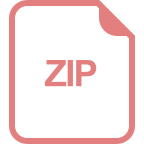














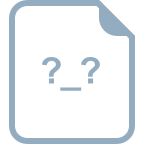
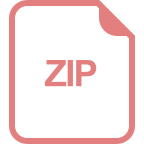