世界杯八强连连看python
时间: 2023-11-21 19:58:04 浏览: 105
以下是世界杯八强连连看Python的实现方法:
1.首先,我们需要将游戏界面的16方格建模为一个存储16个数字的列表,其中每个数字的取值范围为[0,8)且出现两次。可以通过合并两个范围为[0,8)的列表来构建该列表,具体代码如下:
```python
import random
# 生成两个范围为[0,8)的列表
list1 = list(range(8))
list2 = list(range(8))
# 将两个列表合并,并复制一份
num_list = list1 + list2
random.shuffle(num_list)
num_list_copy = num_list[:]
```
2.接下来,我们需要编写游戏主程序的文件。可以将游戏主程序的文件命名为WorldCupTop8.py。在该文件中,我们需要实现鼠标点击事件及功能,具体代码如下:
```python
import pygame
from sys import exit
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption('World Cup Top 8')
# 加载图片
bg_image = pygame.image.load('background.jpg')
num_image = []
for i in range(16):
num_image.append(pygame.image.load(str(i) + '.jpg'))
# 设置字体
font = pygame.font.Font(None, 36)
# 设置游戏状态
SELECT_FIRST = 0
SELECT_SECOND = 1
FINISH = 2
# 初始化游戏状态
game_state = SELECT_FIRST
# 初始化选择的数字
first_num = -1
second_num = -1
# 初始化得分
score = 0
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 处理鼠标点击事件
if event.type == pygame.MOUSEBUTTONDOWN:
if game_state == FINISH:
# 如果游戏已结束,点击鼠标重新开始游戏
num_list = num_list_copy[:]
random.shuffle(num_list)
game_state = SELECT_FIRST
score = 0
elif game_state == SELECT_FIRST:
# 如果是第一次选择数字,记录选择的数字
x, y = pygame.mouse.get_pos()
first_num = x // 100 + (y // 100) * 4
game_state = SELECT_SECOND
elif game_state == SELECT_SECOND:
# 如果是第二次选择数字,记录选择的数字,并判断是否匹配
x, y = pygame.mouse.get_pos()
second_num = x // 100 + (y // 100) * 4
if second_num == first_num:
# 如果选择了同一个数字,重新选择
game_state = SELECT_FIRST
elif num_list[first_num] != num_list[second_num]:
# 如果选择的两个数字不匹配,重新选择
game_state = SELECT_FIRST
else:
# 如果选择的两个数字匹配,得分加1,并检查是否游戏结束
score += 1
if score == 8:
game_state = FINISH
# 绘制背景
screen.blit(bg_image, (0, 0))
# 绘制数字
for i in range(16):
if game_state == FINISH or i == first_num or i == second_num:
# 如果游戏已结束或者该数字已被选择,显示数字图片
screen.blit(num_image[num_list[i]], (i % 4 * 100, i // 4 * 100))
else:
# 如果游戏未结束且该数字未被选择,显示背景图片
screen.blit(bg_image, (i % 4 * 100, i // 4 * 100), (i % 4 * 100, i // 4 * 100, 100, 100))
# 绘制得分
score_text = font.render('Score: ' + str(score), True, (255, 255, 255))
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.update()
```
阅读全文
相关推荐
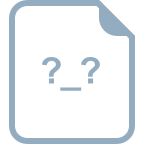
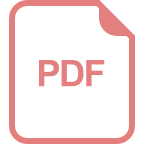




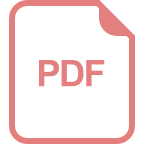
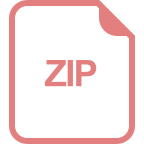
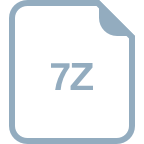
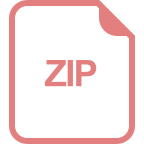
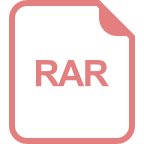
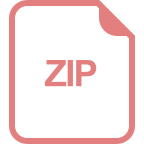
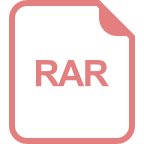

