请问ffmpeg录制MP4文件的时候视频会加速播放,但是音频播放是正常的 c++
时间: 2024-04-10 07:33:48 浏览: 244
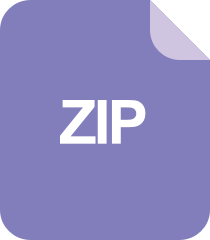
基于C++与ffmpeg的视频播放项目源码(支持本地视频、RTSP流播放,支持录制、OSD叠加)+详细注释.zip
在使用FFmpeg库进行视频录制时,你可以通过设置AVStream的time_base和AVPacket的pts字段来控制视频的播放速度。以下是一个简单的C++示例代码:
```cpp
extern "C" {
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
}
int main() {
// 注册所有的FFmpeg组件
av_register_all();
// 创建AVFormatContext对象
AVFormatContext* formatCtx = nullptr;
avformat_alloc_output_context2(&formatCtx, nullptr, nullptr, "output.mp4");
// 创建视频流和音频流
AVStream* videoStream = avformat_new_stream(formatCtx, nullptr);
AVStream* audioStream = avformat_new_stream(formatCtx, nullptr);
// 设置视频流的time_base,控制播放速度
videoStream->time_base = (AVRational){1, 2}; // 播放速度变为原来的两倍
// 打开输出文件
AVOutputFormat* outputFormat = formatCtx->oformat;
if (avio_open(&formatCtx->pb, "output.mp4", AVIO_FLAG_WRITE) < 0) {
return -1;
}
// 写入文件头
avformat_write_header(formatCtx, nullptr);
// 循环录制视频和音频帧(假设有视频帧和音频帧的数据)
AVPacket pkt;
for (;;) {
// 填充视频帧数据到pkt
av_init_packet(&pkt);
pkt.data = videoFrameData;
pkt.size = videoFrameSize;
pkt.stream_index = videoStream->index;
pkt.pts = av_rescale_q(videoFramePts, (AVRational){1, videoStream->r_frame_rate.den}, videoStream->time_base);
pkt.dts = pkt.pts;
pkt.duration = av_rescale_q(1, (AVRational){1, videoStream->r_frame_rate.den}, videoStream->time_base);
// 写入视频帧数据到输出文件
av_interleaved_write_frame(formatCtx, &pkt);
// 填充音频帧数据到pkt
av_init_packet(&pkt);
pkt.data = audioFrameData;
pkt.size = audioFrameSize;
pkt.stream_index = audioStream->index;
// 写入音频帧数据到输出文件
av_interleaved_write_frame(formatCtx, &pkt);
}
// 写入文件尾
av_write_trailer(formatCtx);
// 释放资源
avio_close(formatCtx->pb);
avformat_free_context(formatCtx);
return 0;
}
```
上述代码仅是一个简单示例,实际应用中需要根据具体情况进行修改和完善。同时,需要自行获取视频帧和音频帧的数据,以及对其进行编码和处理。
阅读全文
相关推荐
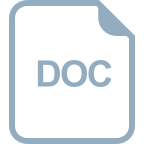
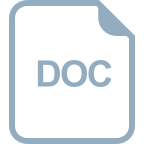
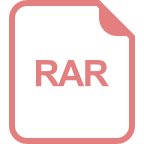
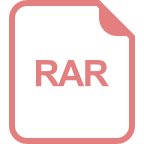
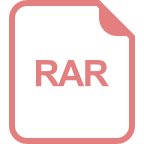
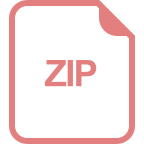
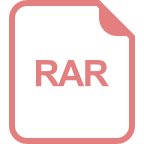
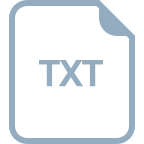
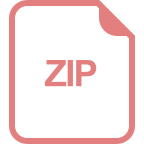
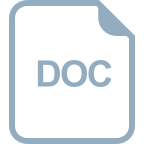
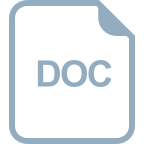
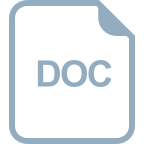





