线性表在单链表中的9种基本运算的算法的python程序实现
时间: 2024-09-18 22:14:37 浏览: 53
线性表在单链表中的基本操作主要包括插入、删除、查找、排序等。这里我将提供Python语言实现这九种常见操作的简短示例:
1. 插入元素 (在链表头部、尾部以及指定位置)
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
def insert_at_head(head, data):
new_node = Node(data)
new_node.next = head
return new_node
def insert_at_tail(head, data):
if not head:
return Node(data)
curr = head
while curr.next:
curr = curr.next
curr.next = Node(data)
return head
def insert_at_position(head, data, position):
if position == 0:
return insert_at_head(head, data)
curr = head
for _ in range(position - 1):
if not curr.next:
break
curr = curr.next
if not curr.next:
curr.next = Node(data)
else:
curr.next = Node(data, curr.next)
return head
```
2. 删除元素 (删除头节点、尾节点以及指定位置的节点)
```python
def delete_at_head(head):
if not head or not head.next:
return head
temp = head.next
head.next = temp.next
return temp if temp else None
def delete_at_tail(head):
if not head:
return head
curr = head
while curr.next and curr.next.next:
curr = curr.next
curr.next = None
return head
def delete_at_position(head, position):
if position == 0:
return delete_at_head(head)
curr = head
count = 0
while count < position - 1 and curr.next:
curr = curr.next
count += 1
if not curr.next:
return head
curr.next = curr.next.next
return head
```
3. 查找元素 (顺序查找)
```python
def search(head, data):
curr = head
while curr:
if curr.data == data:
return True
curr = curr.next
return False
```
4. 其他操作如合并两个已排序链表、反转链表、检查是否有环等,由于篇幅原因,不再逐一列出。
阅读全文
相关推荐
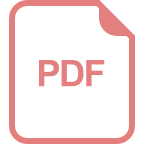
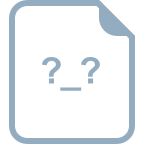
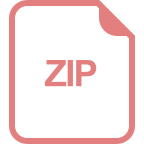



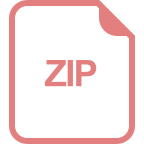
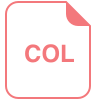
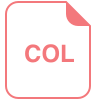
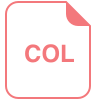








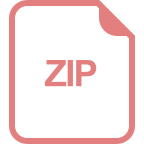