线性表在单链表中的9种基本运算的算法的程序实现
时间: 2024-09-18 12:14:35 浏览: 44
线性表在单链表中的九种基本操作通常包括插入、删除、查找、初始化、长度计算、添加元素到特定位置、移除第一个元素、反转链表以及合并两个有序链表。以下是简化的伪代码描述:
1. **插入(Insertion)**:
```python
void insert_at_start(list *head, int value) {
Node* newNode = new Node;
newNode->data = value;
newNode->next = head;
head = newNode;
}
// 同理,可以编写其他位置(如末尾或指定索引)的插入函数
```
2. **删除(Deletion)**:
```python
void delete_node(Node **head, int key) {
if (head == NULL || (*head)->data == key) {
Node* temp = *head;
*head = (*head)->next;
delete temp;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data != key) {
current = current->next;
}
if (current->next != NULL) {
current->next = current->next->next;
delete current->next;
}
}
}
```
3. **查找(Search)**:
```python
bool search(Node* head, int key) {
Node* current = head;
while (current != NULL) {
if (current->data == key) return true;
current = current->next;
}
return false;
}
```
4. **初始化(Initialization)**:
```c++
list initialize() {
list head = NULL;
return head;
}
```
5. **长度计算(Length Calculation)**:
```c++
int length(list head) {
int count = 0;
Node* current = head;
while (current != NULL) {
count++;
current = current->next;
}
return count;
}
```
6. **添加元素到特定位置(Insert at Position)**:
```c++
void insert_at_position(list *head, int position, int value) {
// 先移动节点至目标位置,然后插入
// ...
}
```
7. **移除第一个元素(Remove First Element)**:
类似于删除,但注意处理头节点的情况。
8. **反转链表(Reversal)**:
```c++
list reverse(list head) {
Node* prev = NULL;
Node* current = head;
Node* nextTemp;
while (current != NULL) {
nextTemp = current->next;
current->next = prev;
prev = current;
current = nextTemp;
}
return prev;
}
```
9. **合并两个有序链表(Merge Sorted Lists)**:
```c++
list merge_sorted_lists(list head1, list head2) {
// ... 实现合并逻辑
}
```
每个操作的具体实现会因语言和数据结构的不同而略有差异,上述代码给出的是通用的思路。如果你需要具体的编程语言版本的代码,比如C++或Python,请告诉我。
阅读全文
相关推荐


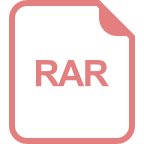















