线性表中的顺序表的9种基本运算的算法的程序实现和线性表在单链表中的9种基本运算的算法的程序实现。
时间: 2024-09-18 11:14:13 浏览: 10
顺序表的基本操作通常包括插入、删除、查找、排序等,以下是部分基本运算的简单算法实现:
**顺序表(数组)的九种基本运算:**
1. **初始化(创建空表):**
```c++
void createList(int n) {
list = new int[n];
length = 0;
}
```
2. **插入元素:**
```c++
void insertAtBegin(int value) {
if (length == capacity) expand();
list[length++] = value;
}
```
3. **删除元素:**
```c++
void deleteElement(int position) {
if (position < 0 || position >= length) return;
for (int i = position; i < length - 1; i++) {
list[i] = list[i + 1];
}
length--;
}
```
4. **查找元素:**
```c++
int search(int value) {
for (int i = 0; i < length; i++) {
if (list[i] == value) return i;
}
return -1;
}
```
**单链表的九种基本运算:**
1. **初始化(创建空链表):**
```c++
struct Node* createList() {
head = NULL;
tail = NULL;
return NULL;
}
```
2. **插入元素:**
```c++
void insertAtBegin(struct Node* &head, int value) {
struct Node* newNode = new Node{value, head};
if (head != NULL) head->prev = newNode;
head = newNode;
}
```
3. **删除元素:**
```c++
void deleteElement(struct Node* &head, int value) {
struct Node* current = head;
while (current != NULL && current->data != value) {
current = current->next;
}
if (current != NULL) {
if (current->prev != NULL) current->prev->next = current->next;
else head = current->next;
delete current;
}
}
```
4. **查找元素:**
```c++
struct Node* search(struct Node* head, int value) {
struct Node* current = head;
while (current != NULL) {
if (current->data == value) return current;
current = current->next;
}
return NULL;
}
```
相关推荐
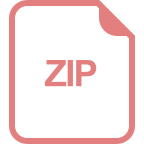
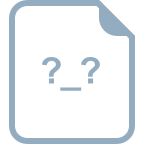
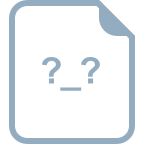














