std::string格式如何用log_e输出
时间: 2023-05-04 07:05:43 浏览: 69
std::string是C++ STL库中的一个类,用于表示可变字符串。要将std::string输出到日志中(例如使用log_e函数),可以使用其c_str()成员函数,该函数以C字符串的形式返回一个指向std::string对象内部数据的指针。
例如,以下代码展示了如何将std::string格式的消息输出到日志中:
```c++
#include <string>
#include <android/log.h>
void log_error(const std::string& msg) {
const char* tag = "MyTag";
__android_log_write(ANDROID_LOG_ERROR, tag, msg.c_str());
}
```
在该代码中,我们定义了一个名为log_error的函数,它使用__android_log_write函数将std::string消息作为一个C字符串写入Android日志。由于msg参数是一个const std::string的引用,我们可以通过msg.c_str()调用获得其C字符串表示形式。此外,我们指定日志级别为ANDROID_LOG_ERROR,并指定了输出消息的标记为"MyTag"。
相关问题
#include<iostream> #include<ctime> #include<chrono> #include<string> #include<filesystem> #include<fstream> #include<sstream> #include<thread> #include<boost/filesystem.hpp> const uintmax_t MAX_LOGS_SIZE = 10ull * 1024ull * 1024ull * 1024ull; //const uintmax_t MAX_LOGS_SIZE = 10ull; void create_folder(std::string folder_name) { boost::filesystem::create_directory(folder_name); std::string sub_foldername=folder_name+"/logs_ros"; boost::filesystem::create_directory(sub_foldername); } std::string get_current_time() { auto now = std::chrono::system_clock::now(); std::time_t now_c = std::chrono::system_clock::to_time_t(now); std::tm parts = *std::localtime(&now_c); char buffer[20]; std::strftime(buffer, sizeof(buffer), "%Y-%m-%d-%H-%M", &parts); return buffer; } void check_logs_size() { std::string logs_path = "/home/sage/logs/"; boost::filesystem::path logs_dir(logs_path); std::uintmax_t total_size = 0; for (const auto& file : boost::filesystem::recursive_directory_iterator(logs_dir)) { if (boost::filesystem::is_regular_file(file)) { total_size += boost::filesystem::file_size(file); } } if (total_size > MAX_LOGS_SIZE) { boost::filesystem::path earliest_dir; std::time_t earliest_time = std::time(nullptr); for (const auto& dir : boost::filesystem::directory_iterator(logs_dir)) { if (boost::filesystem::is_directory(dir)) { std::string dir_name = dir.path().filename().string(); std::tm time_parts = {}; std::istringstream ss(dir_name); std::string part; std::getline(ss, part, '-'); time_parts.tm_year = std::stoi(part) - 1900; std::getline(ss, part, '-'); time_parts.tm_mon = std::stoi(part) - 1; std::getline(ss, part, '-'); time_parts.tm_mday = std::stoi(part); std::getline(ss, part, '-'); time_parts.tm_hour = std::stoi(part); std::getline(ss, part, '-'); time_parts.tm_min = std::stoi(part); std::time_t dir_time = std::mktime(&time_parts); if (dir_time < earliest_time) { earliest_time = dir_time; earliest_dir = dir.path(); } } } if (!earliest_dir.empty()) { boost::filesystem::remove_all(earliest_dir); } } } int main() { std::string logs_path = "/home/sage/logs/"; while (true) { std::chrono::system_clock::time_point now = std::chrono::system_clock::now(); std::time_t now_c = std::chrono::system_clock::to_time_t(now); std::tm parts = *std::localtime(&now_c); if (parts.tm_min % 10 == 0) { std::string folder_name = logs_path + get_current_time(); create_folder(folder_name); } check_logs_size(); std::this_thread::sleep_for(std::chrono::minutes(1)); } return 0; }修改为ros节点
好的,我可以帮您将这段代码修改为ROS节点。首先,我们需要包含ROS所需的头文件,例如:
```cpp
#include <ros/ros.h>
```
然后,我们需要在main函数中初始化ROS节点:
```cpp
int main(int argc, char** argv) {
ros::init(argc, argv, "log_manager_node");
ros::NodeHandle nh;
// ...
return 0;
}
```
接下来,我们需要将定时任务和日志删除任务分别封装为ROS的定时器和服务。在main函数中,我们可以这样定义一个定时器:
```cpp
ros::Timer timer = nh.createTimer(ros::Duration(60.0), timerCallback);
```
其中,`timerCallback` 是一个你需要定义的回调函数,它将在每隔60秒被定时器调用一次。在回调函数中,你可以像之前的代码一样执行创建文件夹和检查日志大小的任务。
同样地,我们可以定义一个ROS服务来执行删除任务。我们需要在main函数中定义一个服务服务器:
```cpp
ros::ServiceServer delete_logs_server = nh.advertiseService("delete_logs", deleteLogsCallback);
```
其中,`deleteLogsCallback` 是一个你需要定义的服务回调函数,它将在收到删除日志服务请求时被调用。在回调函数中,你可以像之前的代码一样执行删除日志的任务。
最后,完整的ROS节点代码如下:
```cpp
#include <ros/ros.h>
#include <ctime>
#include <chrono>
#include <string>
#include <filesystem>
#include <fstream>
#include <sstream>
#include <thread>
#include <boost/filesystem.hpp>
const uintmax_t MAX_LOGS_SIZE = 10ull * 1024ull * 1024ull * 1024ull;
void create_folder(std::string folder_name) {
boost::filesystem::create_directory(folder_name);
std::string sub_foldername = folder_name + "/logs_ros";
boost::filesystem::create_directory(sub_foldername);
}
std::string get_current_time() {
auto now = std::chrono::system_clock::now();
std::time_t now_c = std::chrono::system_clock::to_time_t(now);
std::tm parts = *std::localtime(&now_c);
char buffer[20];
std::strftime(buffer, sizeof(buffer), "%Y-%m-%d-%H-%M", &parts);
return buffer;
}
void check_logs_size() {
std::string logs_path = "/home/sage/logs/";
boost::filesystem::path logs_dir(logs_path);
std::uintmax_t total_size = 0;
for (const auto& file : boost::filesystem::recursive_directory_iterator(logs_dir)) {
if (boost::filesystem::is_regular_file(file)) {
total_size += boost::filesystem::file_size(file);
}
}
if (total_size > MAX_LOGS_SIZE) {
boost::filesystem::path earliest_dir;
std::time_t earliest_time = std::time(nullptr);
for (const auto& dir : boost::filesystem::directory_iterator(logs_dir)) {
if (boost::filesystem::is_directory(dir)) {
std::string dir_name = dir.path().filename().string();
std::tm time_parts = {};
std::istringstream ss(dir_name);
std::string part;
std::getline(ss, part, '-');
time_parts.tm_year = std::stoi(part) - 1900;
std::getline(ss, part, '-');
time_parts.tm_mon = std::stoi(part) - 1;
std::getline(ss, part, '-');
time_parts.tm_mday = std::stoi(part);
std::getline(ss, part, '-');
time_parts.tm_hour = std::stoi(part);
std::getline(ss, part, '-');
time_parts.tm_min = std::stoi(part);
std::time_t dir_time = std::mktime(&time_parts);
if (dir_time < earliest_time) {
earliest_time = dir_time;
earliest_dir = dir.path();
}
}
}
if (!earliest_dir.empty()) {
boost::filesystem::remove_all(earliest_dir);
}
}
}
void timerCallback(const ros::TimerEvent& event) {
std::string logs_path = "/home/sage/logs/";
std::chrono::system_clock::time_point now = std::chrono::system_clock::now();
std::time_t now_c = std::chrono::system_clock::to_time_t(now);
std::tm parts = *std::localtime(&now_c);
if (parts.tm_min % 10 == 0) {
std::string folder_name = logs_path + get_current_time();
create_folder(folder_name);
}
check_logs_size();
}
bool deleteLogsCallback(std_srvs::Empty::Request& req, std_srvs::Empty::Response& res) {
std::string logs_path = "/home/sage/logs/";
boost::filesystem::path logs_dir(logs_path);
boost::filesystem::remove_all(logs_dir);
return true;
}
int main(int argc, char** argv) {
ros::init(argc, argv, "log_manager_node");
ros::NodeHandle nh;
ros::Timer timer = nh.createTimer(ros::Duration(60.0), timerCallback);
ros::ServiceServer delete_logs_server = nh.advertiseService("delete_logs", deleteLogsCallback);
ros::spin();
return 0;
}
```
在这个节点中,我们定义了一个名为 `log_manager_node` 的ROS节点,并在其中实现了一个定时器和一个服务。定时器每隔60秒检查一次日志大小并创建新的日志文件夹,服务则用于删除所有日志文件。
orange.cpp:120:60: error: cannot convert ‘__gnu_cxx::__alloc_traits<std::allocator<std::__cxx11::basic_string<char> >, std::__cxx11::basic_string<char> >::value_type’ {aka ‘std::__cxx11::basic_string<char>’} to ‘const char*’ strcpy(PrintLog[index].cpValue,strResult.back());
这个错误是由于`strcpy`函数的参数类型不匹配造成的。`strcpy`函数的第一个参数应该是`char*`类型,而你传入的是`std::__cxx11::basic_string<char>`类型。你可以使用`std::strcpy`来解决这个问题,或者使用`std::string`的成员函数`c_str()`来获取一个以null结尾的C风格字符串,然后再进行复制操作。示例代码如下:
```cpp
strcpy(PrintLog[index].cpValue, strResult.back().c_str());
```
请确保`PrintLog[index].cpValue`有足够的空间来存储复制后的字符串,以避免缓冲区溢出的风险。
相关推荐
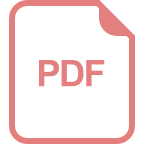
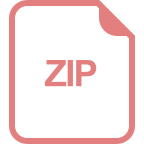
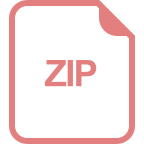











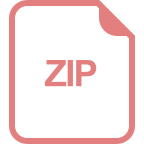
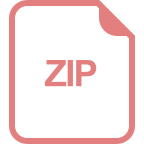