coap协议C语言实现
时间: 2023-11-26 10:06:13 浏览: 129
CoAP(Constrained Application Protocol)是一种轻量级的应用层协议,用于在受限的网络环境中传输数据。以下是一个简单的CoAP协议C语言实现的示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#include <arpa/inet.h>
#define COAP_VERSION 1
#define COAP_HEADER_LENGTH 4
typedef struct {
uint8_t version;
uint8_t type;
uint8_t token_length;
uint8_t code;
uint16_t message_id;
} coap_header_t;
typedef struct {
uint8_t *token;
uint16_t token_length;
uint8_t *payload;
uint16_t payload_length;
} coap_message_t;
typedef struct {
int socket_fd;
struct sockaddr_in address;
} coap_endpoint_t;
typedef struct {
uint8_t *data;
uint16_t length;
} coap_buffer_t;
coap_header_t *coap_create_header(uint8_t type, uint8_t code, uint16_t message_id) {
coap_header_t *header = calloc(1, sizeof(coap_header_t));
header->version = COAP_VERSION;
header->type = type;
header->token_length = 0;
header->code = code;
header->message_id = htons(message_id);
return header;
}
void coap_free_header(coap_header_t *header) {
free(header);
}
coap_message_t *coap_create_message(uint8_t type, uint8_t code, uint16_t message_id) {
coap_message_t *message = calloc(1, sizeof(coap_message_t));
message->token = NULL;
message->token_length = 0;
message->payload = NULL;
message->payload_length = 0;
coap_header_t *header = coap_create_header(type, code, message_id);
message->payload_length += COAP_HEADER_LENGTH;
message->payload = calloc(1, message->payload_length);
memcpy(message->payload, header, COAP_HEADER_LENGTH);
coap_free_header(header);
return message;
}
void coap_free_message(coap_message_t *message) {
free(message->token);
free(message->payload);
free(message);
}
coap_endpoint_t *coap_create_endpoint(char *ip, int port) {
coap_endpoint_t *endpoint = calloc(1, sizeof(coap_endpoint_t));
endpoint->socket_fd = socket(AF_INET, SOCK_DGRAM, IPPROTO_UDP);
memset(&endpoint->address, 0, sizeof(endpoint->address));
endpoint->address.sin_family = AF_INET;
endpoint->address.sin_addr.s_addr = inet_addr(ip);
endpoint->address.sin_port = htons(port);
return endpoint;
}
void coap_free_endpoint(coap_endpoint_t *endpoint) {
close(endpoint->socket_fd);
free(endpoint);
}
coap_buffer_t *coap_receive(coap_endpoint_t *endpoint) {
coap_buffer_t *buffer = calloc(1, sizeof(coap_buffer_t));
socklen_t address_length = sizeof(endpoint->address);
int length = recvfrom(endpoint->socket_fd, buffer->data, buffer->length, 0, (struct sockaddr *) &endpoint->address, &address_length);
buffer->length = length;
return buffer;
}
void coap_send(coap_endpoint_t *endpoint, coap_message_t *message) {
sendto(endpoint->socket_fd, message->payload, message->payload_length, 0, (struct sockaddr *) &endpoint->address, sizeof(endpoint->address));
}
int main() {
coap_endpoint_t *endpoint = coap_create_endpoint("127.0.0.1", 5683);
coap_message_t *message = coap_create_message(0, 1, 1);
coap_send(endpoint, message);
coap_buffer_t *buffer = coap_receive(endpoint);
printf("Received %d bytes: %.*s\n", buffer->length, buffer->length, buffer->data);
coap_free_message(message);
coap_free_endpoint(endpoint);
free(buffer);
return 0;
}
```
这个示例实现了CoAP协议的基本功能,包括创建和发送CoAP消息,以及接收和处理CoAP消息。在这个示例中,我们使用了标准的C语言库函数和一些网络相关的函数来实现CoAP协议的功能。如果您想要了解更多关于CoAP协议的信息,请参考CoAP协议的官方网站。
相关推荐
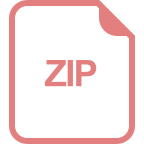
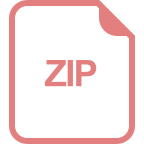














