基于springboot设计能接入 http mqtt coap协议的物联网平台,写出示范代码
时间: 2024-02-01 18:14:10 浏览: 166
以下是一个基于Spring Boot设计的物联网平台示范代码,可以接入HTTP、MQTT以及CoAP协议:
```java
@RestController
public class DeviceController {
@Autowired
private DeviceService deviceService;
// HTTP接口示例
@PostMapping("/devices")
public ResponseEntity<?> addDevice(@RequestBody Device device) {
deviceService.addDevice(device);
return ResponseEntity.ok().build();
}
// MQTT接口示例
@SneakyThrows
@PostMapping("/mqtt/devices")
public ResponseEntity<?> addDeviceMqtt(@RequestBody Device device) {
MqttClient client = new MqttClient("tcp://localhost:1883", "client-id");
client.connect();
String topic = "devices";
String payload = new ObjectMapper().writeValueAsString(device);
MqttMessage message = new MqttMessage(payload.getBytes());
client.publish(topic, message);
return ResponseEntity.ok().build();
}
// CoAP接口示例
@PostMapping("/coap/devices")
public ResponseEntity<?> addDeviceCoap(@RequestBody Device device) {
CoapClient client = new CoapClient("coap://localhost/devices");
CoapResponse response = client.post(new Gson().toJson(device), MediaTypeRegistry.APPLICATION_JSON);
if (response.isSuccess()) {
return ResponseEntity.ok().build();
}
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
```
其中,`DeviceService`是一个设备管理的服务类,提供了添加、查询、删除等设备管理功能。在这个示例中,我们演示了如何通过HTTP、MQTT以及CoAP协议来调用这个服务类的方法。
由于涉及到MQTT和CoAP协议的接入,我们需要添加相应的依赖:
```xml
<dependency>
<groupId>org.eclipse.paho</groupId>
<artifactId>org.eclipse.paho.client.mqttv3</artifactId>
<version>1.2.5</version>
</dependency>
<dependency>
<groupId>com.sksamuel.jersey</groupId>
<artifactId>jersey-coap</artifactId>
<version>3.0.2</version>
</dependency>
```
在`application.properties`中配置MQTT和CoAP的端口:
```properties
spring.mqtt.port=1883
spring.coap.port=5683
```
以上示例代码可以让我们了解如何在Spring Boot中接入不同的物联网协议,同时也可以作为一个基础架构,进一步扩展业务功能。
阅读全文
相关推荐
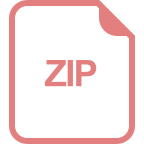
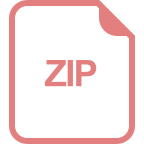
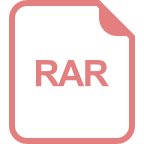















