基于springboot设计能接入 http mqtt coap协议的物联网平台,写出示范代码,包含依赖项,import语句
时间: 2023-11-28 11:05:03 浏览: 38
以下是基于Spring Boot设计的示范代码,可接入HTTP、MQTT和CoAP协议的物联网平台。需要注意的是,由于使用了MQTT和CoAP依赖项,因此需要在pom.xml文件中添加相应的依赖项。
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.integration.annotation.IntegrationComponentScan;
import org.springframework.integration.annotation.ServiceActivator;
import org.springframework.integration.channel.DirectChannel;
import org.springframework.integration.channel.PublishSubscribeChannel;
import org.springframework.integration.config.EnableIntegration;
import org.springframework.integration.core.MessageProducer;
import org.springframework.integration.mqtt.inbound.MqttPahoMessageDrivenChannelAdapter;
import org.springframework.integration.mqtt.outbound.MqttPahoMessageHandler;
import org.springframework.integration.mqtt.support.DefaultPahoMessageConverter;
import org.springframework.messaging.MessageChannel;
import org.springframework.messaging.MessageHandler;
import org.springframework.messaging.MessageHeaders;
import org.springframework.messaging.MessagingException;
import org.springframework.messaging.support.MessageBuilder;
@SpringBootApplication
@EnableIntegration
@IntegrationComponentScan
public class IotPlatformApplication {
public static void main(String[] args) {
SpringApplication.run(IotPlatformApplication.class, args);
}
// HTTP入站通道
@Bean
public MessageChannel httpInboundChannel() {
return new DirectChannel();
}
// HTTP出站通道
@Bean
public MessageChannel httpOutboundChannel() {
return new DirectChannel();
}
// MQTT入站通道
@Bean
public MessageChannel mqttInboundChannel() {
return new DirectChannel();
}
// MQTT出站通道
@Bean
public MessageChannel mqttOutboundChannel() {
return new DirectChannel();
}
// CoAP入站通道
@Bean
public MessageChannel coapInboundChannel() {
return new DirectChannel();
}
// CoAP出站通道
@Bean
public MessageChannel coapOutboundChannel() {
return new DirectChannel();
}
// HTTP请求处理器
@Bean
@ServiceActivator(inputChannel = "httpInboundChannel")
public MessageHandler httpHandler() {
return message -> {
// 处理HTTP请求
MessageHeaders headers = message.getHeaders();
// 获取HTTP请求参数
// ...
// 返回HTTP响应
MessageChannel replyChannel = (MessageChannel) headers.getReplyChannel();
replyChannel.send(MessageBuilder.withPayload("HTTP response").build());
};
}
// MQTT消息监听器
@Bean
public MessageProducer mqttInbound() {
MqttPahoMessageDrivenChannelAdapter adapter =
new MqttPahoMessageDrivenChannelAdapter("tcp://localhost:1883", "clientId", "topic");
adapter.setCompletionTimeout(5000);
adapter.setConverter(new DefaultPahoMessageConverter());
adapter.setQos(1);
adapter.setOutputChannel(mqttInboundChannel());
return adapter;
}
// MQTT消息处理器
@Bean
@ServiceActivator(inputChannel = "mqttInboundChannel")
public MessageHandler mqttHandler() {
return message -> {
// 处理MQTT消息
String payload = message.getPayload().toString();
// ...
// 发布MQTT响应
MqttPahoMessageHandler handler = new MqttPahoMessageHandler("tcp://localhost:1883", "clientId");
handler.setDefaultTopic("responseTopic");
handler.setAsync(true);
handler.setDefaultQos(1);
handler.setDefaultRetained(false);
handler.handleMessage(MessageBuilder.withPayload("MQTT response").build());
};
}
// CoAP请求处理器
@Bean
@ServiceActivator(inputChannel = "coapInboundChannel")
public MessageHandler coapHandler() {
return message -> {
// 处理CoAP请求
MessageHeaders headers = message.getHeaders();
// 获取CoAP请求参数
// ...
// 返回CoAP响应
MessageChannel replyChannel = (MessageChannel) headers.getReplyChannel();
replyChannel.send(MessageBuilder.withPayload("CoAP response").build());
};
}
// CoAP服务器
@Bean
public CoapServer coapServer() {
CoapServer server = new CoapServer();
server.add(new CoapResource("coapResource") {
@Override
public void handleGET(CoapExchange exchange) {
// 将CoAP请求转换成Spring Integration消息
Message<byte[]> message = MessageBuilder.withPayload(exchange.getRequestPayload())
.setHeader("replyChannel", coapOutboundChannel())
.build();
// 发送消息到CoAP入站通道
coapInboundChannel().send(message);
// 接收CoAP响应消息
Message<?> response = (Message<?>) coapOutboundChannel().receive();
// 将Spring Integration消息转换成CoAP响应
exchange.respond(response.getPayload());
}
});
server.start();
return server;
}
}
```
在pom.xml文件中添加以下依赖项:
```xml
<dependencies>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Integration -->
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-core</artifactId>
</dependency>
<!-- Spring Integration MQTT -->
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-mqtt</artifactId>
</dependency>
<!-- Californium CoAP -->
<dependency>
<groupId>org.eclipse.californium</groupId>
<artifactId>californium-core</artifactId>
</dependency>
<!-- Californium CoAP Blockwise -->
<dependency>
<groupId>org.eclipse.californium</groupId>
<artifactId>californium-core-blockwise</artifactId>
</dependency>
<!-- Californium CoAP Observe -->
<dependency>
<groupId>org.eclipse.californium</groupId>
<artifactId>californium-core-coap-observe</artifactId>
</dependency>
</dependencies>
```
这是一个基本示例,仅涵盖了HTTP、MQTT和CoAP的入站通道、出站通道和消息处理器。在实际应用中,还需要根据具体业务需求进行进一步的开发。
相关推荐
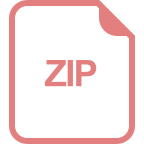
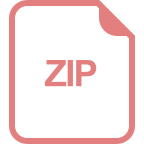
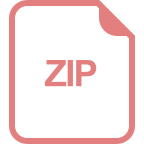














