哲学家进餐问题pv代码
时间: 2023-11-12 18:21:12 浏览: 63
哲学家进餐问题是一个经典的同步问题,可以用多种方式实现。以下是一个使用pv操作的示例代码:
```python
import threading
import time
class Philosopher(threading.Thread):
def __init__(self, name, left_fork, right_fork, sem):
threading.Thread.__init__(self)
self.name = name
self.left_fork = left_fork
self.right_fork = right_fork
self.sem = sem
def run(self):
while True:
# 每个哲学家思考一段时间
print(f"{self.name} is thinking.")
time.sleep(2)
# 尝试获取左边的叉子,如果被占用就等待
print(f"{self.name} is hungry and wants to eat.")
self.sem.acquire()
self.left_fork.acquire()
print(f"{self.name} got the left fork.")
# 尝试获取右边的叉子,如果被占用就等待
self.right_fork.acquire()
print(f"{self.name} got the right fork and starts eating.")
time.sleep(3)
# 放下叉子,释放信号量
self.left_fork.release()
self.right_fork.release()
self.sem.release()
print(f"{self.name} finished eating and put down forks.")
if __name__ == '__main__':
# 创建5个叉子
forks = [threading.Lock() for n in range(5)]
# 创建5个哲学家,每个哲学家拥有左右两个叉子
philosophers = [Philosopher(f"Philosopher {n}", forks[n], forks[(n+1)%5], threading.Semaphore(4)) for n in range(5)]
# 启动每个哲学家的线程
for p in philosophers:
p.start()
# 等待所有哲学家线程结束
for p in philosophers:
p.join()
```
在这个例子中,每个哲学家被表示为一个线程,每个叉子被表示为一个锁。为了避免死锁,我们使用了一个信号量来限制同时进餐的哲学家数量。每个哲学家循环执行思考、尝试获取叉子、进餐、放下叉子等操作。整个程序在所有哲学家线程结束之后退出。
相关推荐
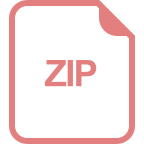
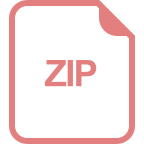







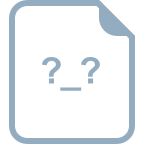
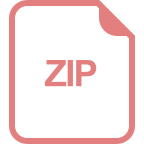
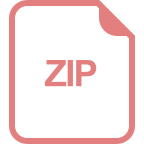