蒙特卡洛树搜索python代码
时间: 2024-10-15 11:13:40 浏览: 42
蒙特卡洛树搜索(Monte Carlo Tree Search,简称MCTS)是一种常用的决策过程优化算法,在游戏策略、人工智能等领域广泛应用。在Python中,你可以利用如`uctlib`这样的库来实现基本的MCTS。以下是一个简单的示例:
```python
import random
# 假设这是一个游戏环境,比如棋盘游戏
class GameEnvironment:
def get_action_probabilities(self, state):
# 根据当前状态计算所有可行动作的概率
pass
def simulate(self, action):
# 模拟一次游戏,返回结果(胜、负或平局)
pass
class MCTSPlayer:
def __init__(self, environment, n_simulations=1000):
self.environment = environment
self.n_simulations = n_simulations
self.root = None
def select_node(self, node):
# 选择节点函数,通常基于UCT公式(Upper Confidence Bound for Trees)
...
def expand_node(self, node):
# 扩展节点,生成新节点并添加到树中
...
def backup(self, leaf_value):
# 回溯更新节点价值
...
def run_mcts(self, state):
root = Node(state)
for _ in range(self.n_simulations):
node = self.select_node(root)
if node.is_leaf():
result = self.environment.simulate(node.action)
node.value = result
self.backup(node.value)
else:
child = self.expand_node(node)
result = self.environment.simulate(child.action)
child.value = result
self.backup(result)
return max(root.children, key=lambda x: x.value).action
```
在这个例子中,你需要实现`select_node`、`expand_node`和`backup`方法,并在`GameEnvironment`中定义如何获取概率和模拟游戏。注意这只是一个基础版本,实际应用中可能会更复杂,例如加入温度衰减等优化。
阅读全文
相关推荐
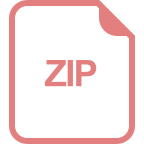
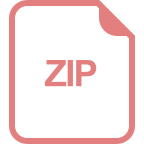
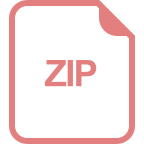
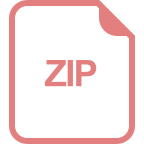
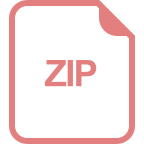
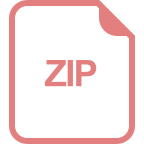
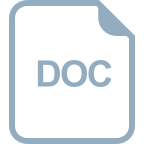
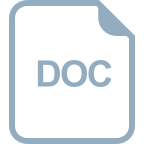
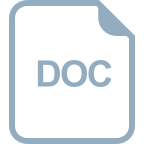
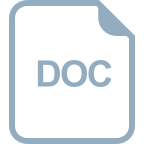
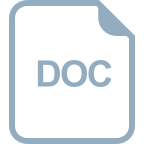
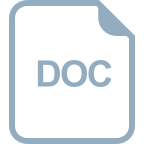
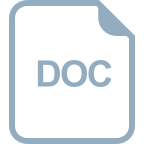
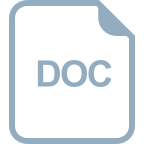




