如何使用panda3d实现对话系统?详细说明步骤,并给出一个简单的demo。
时间: 2023-03-15 21:25:56 浏览: 78
使用Panda3D来实现对话系统具体步骤如下:1.准备好所需要的资源,包括3D模型、文本文件、声音文件等;2.使用Panda3D的GUI模块创建一个GUI管理器;3.创建一个对话系统管理器,用于管理对话流程;4.使用Panda3D的对话系统模块,读取文本文件,并为每一个对话结点添加声音文件;5.创建一个3D场景,并将角色放置在场景中;6.创建一个事件管理器,用于处理玩家发起的操作;7.根据玩家的操作更新GUI,并继续播放对话流程;8.在游戏结束时,清理资源,并将结果保存至指定位置。以上就是使用Panda3D实现对话系统的具体步骤,下面是一个简单的demo:import direct.directbase.DirectStart
from direct.showbase.DirectObject import DirectObjectclass Game(DirectObject):
def __init__(self):
self.dialogMgr = DialogMgr()
self.dialogMgr.loadFile("dialog.txt")
self.dialogMgr.start()
game = Game()
base.run()
相关问题
如何使用panda3d制作对话系统?简短回答。
Panda3D是一个开源的3D游戏引擎,它提供了一个基于Python的开发环境,可以轻松地创建游戏和应用程序。要使用Panda3D创建对话系统,你可以遵循以下步骤:
1. 创建一个角色对象,用于扮演对话中的人物角色。
2. 创建一个对话系统管理器,用于控制对话的流程和逻辑。
3. 在对话系统管理器中定义对话流程,包括对话内容、选项和分支。
4. 在游戏中添加一个对话触发器,当玩家接近角色时触发对话。
5. 实现对话框,用于在游戏中显示对话内容和选项。
6. 根据玩家的选择更新对话流程,直到对话结束。
以上是一个简单的对话系统的基本实现步骤,具体实现过程还需要根据你的具体需求和游戏逻辑进行定制化开发。
使用panda3d实现人物对话系统,给出代码。
### 回答1:
首先,您需要使用Panda3D来设置动作和语音:# create an Actor object
from direct.actor.Actor import Actor# load the character
character = Actor("models/panda-model",
{"walk": "models/panda-walk4"})# loop the walk animation
character.loop("walk")# create a TextNode object
from direct.gui.OnscreenText import OnscreenText# create the dialogue
dialogue = OnscreenText(text="你好吗?", pos=(0, 0.5), scale=0.07)# add the dialogue to the scene
character.attachNewNode(dialogue)# create a CollisionSphere object
from direct.showbase.DirectObject import DirectObject# create a collision event
class CollisionEvent(DirectObject):
def __init__(self):
self.accept("collide", self.collide)
def collide(self, entry):
print("Collision detected!")# run the CollisionEvent
collision = CollisionEvent()# add an event to the CollisionSphere
base.cTrav.addCollider(character, base.pusher)# set up the task manager
from direct.task import Task# create a task that checks for collisions
def checkCollision(task):
base.cTrav.traverse(render)
if entry.getIntoNode().getName() == "collide":
dialogue.setText("再见")
else:
dialogue.setText("你好吗?")
return task.cont# add the task to the task manager
taskMgr.add(checkCollision, "checkCollision")
### 回答2:
使用Panda3D可以实现人物对话系统,以下是一个简单的示例代码:
```python
from direct.showbase.ShowBase import ShowBase
from panda3d.core import TextNode, Vec3
from direct.gui.OnscreenText import OnscreenText
class MyGame(ShowBase):
def __init__(self):
ShowBase.__init__(self)
# 创建文本节点
self.text_node = TextNode('dialog')
self.text_node.setText("")
# 将文本节点绑定到3D场景中的一个节点
self.dialog_np = aspect2d.attachNewNode(self.text_node)
self.dialog_np.setScale(0.1)
self.dialog_np.setPos(-1, 0, 0)
# 创建对话框用于显示文本
self.dialog_box = OnscreenText(parent=base.a2dBottomLeft,
text="",
pos=(0.07, 0.1),
scale=0.07,
fg=(1, 1, 1, 1),
align=TextNode.ALeft)
# 设置键盘事件
self.accept("space", self.show_dialog)
def show_dialog(self):
# 假设对话内容为 "Hello, Panda3D!"
dialog_content = "Hello, Panda3D!"
# 显示对话框
self.dialog_box.setText(dialog_content)
# 设置文本节点的内容
self.text_node.setText(dialog_content)
self.text_node.setAlign(TextNode.ALeft)
def run(self):
self.show_dialog()
self.run()
game = MyGame()
game.run()
```
以上代码创建了一个Panda3D游戏的简单示例,按下空格键即可显示一个对话框,对话框中显示文本为"Hello, Panda3D!"。在文本节点中,我们把文本设置为"Hello, Panda3D!",并将其绑定到了2D场景中的一个节点上,以便在游戏中显示对话内容。同时,在屏幕上方显示了对话框,用于更好地呈现对话内容。
### 回答3:
使用panda3d实现人物对话系统需要先导入panda3d库。以下是一个简单的代码示例:
```python
from panda3d.core import TextNode
from direct.showbase.ShowBase import ShowBase
from direct.gui.DirectGui import DirectFrame, DirectButton
class DialogSystem(ShowBase):
def __init__(self):
ShowBase.__init__(self)
# 创建对话框
self.dialog_frame = DirectFrame(frameColor=(0, 0, 0, 0.8),
pos=(-1.1, 0, -0.8),
scale=(1.8, 1, 0.2))
self.dialog_text = DirectLabel(text='',
text_fg=(1, 1, 1, 1),
text_font=self.loader.loadFont('font.ttf'),
frameSize=self.dialog_frame.getBounds(),
parent=self.dialog_frame)
# 创建对话选项按钮
self.option_buttons = []
for idx in range(3):
button = DirectButton(text='',
pos=(-1.15 + idx % 2 * 0.75, 0, -1 + idx // 2 * 0.2),
scale=(0.6, 1, 0.15),
command=self.on_option_selected,
extraArgs=[idx])
self.option_buttons.append(button)
# 初始化对话数据
self.dialog_data = [
{
'speaker': 'Alice',
'text': 'Hello, how are you?',
'options': [
'I am fine, thanks.',
'Not so good.',
'Who are you?'
]
},
# 继续添加其他对话数据...
]
# 开始对话
self.dialog_index = 0
self.show_dialog()
def show_dialog(self):
dialog = self.dialog_data[self.dialog_index]
self.dialog_text['text'] = dialog['text']
for i, option in enumerate(dialog['options']):
self.option_buttons[i]['text'] = option
self.option_buttons[i].show()
speaker = dialog['speaker']
self.speak(speaker)
def on_option_selected(self, option_index):
selected_option = self.dialog_data[self.dialog_index]['options'][option_index]
# 处理选项选择后的逻辑...
# 更新对话索引
self.dialog_index += 1
self.show_dialog()
def speak(self, speaker):
print(speaker + ' is speaking.')
app = DialogSystem()
app.run()
```
这段代码使用panda3d库创建了一个简单的人物对话系统。通过DirectFrame创建了一个对话框,通过DirectButton创建了对话选项按钮,并且使用DirectLabel显示对话文本。对话数据存储在一个列表中,包含了发言者、对话文本和选项内容。使用show_dialog方法显示当前对话数据,并且通过on_option_selected方法处理选项选择的逻辑。可以根据需求进一步扩展和完善该对话系统。
相关推荐
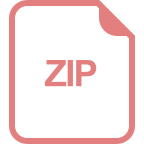
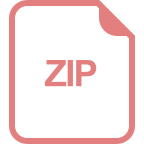
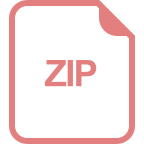












