使用panda3d写一个对话系统。
时间: 2023-03-30 07:01:25 浏览: 153
好的,我可以回答这个问题。Panda3D 是一个强大的游戏引擎,可以用来开发游戏和交互式应用程序。要创建一个对话系统,您可以使用 Panda3D 的内置用户界面库,例如 DirectGUI。您可以创建一个文本框和一个按钮,当用户点击按钮时,将文本框中的文本发送到您的对话系统中进行处理。您可以使用 Python 编写对话系统的逻辑,并使用 Panda3D 的网络模块与服务器进行通信。希望这可以帮助您开始编写您的对话系统。
相关问题
如何使用panda3d写出对话系统
使用 Panda3D 编写对话系统需要以下步骤:
1. 创建一个对话系统的类,该类应该包含对话的所有信息,如对话内容、对话选项等。
2. 在 Panda3D 中创建一个对话框,用于显示对话内容和选项。
3. 在对话框中添加按钮,用于选择对话选项。
4. 在对话框中添加文本框,用于显示对话内容。
5. 在对话框中添加事件处理程序,用于处理对话选项的选择。
6. 在游戏中使用该对话系统类,将对话内容和选项传递给对话框,然后等待用户选择。
以下是一个简单的示例代码:
```python
from direct.showbase.ShowBase import ShowBase
from direct.gui.DirectGui import *
class DialogSystem:
def __init__(self, dialog, options, callback):
self.dialog = dialog
self.options = options
self.callback = callback
def show(self):
self.dialogBox = DirectDialog(text=self.dialog, buttonTextList=self.options, command=self.callback)
class MyApp(ShowBase):
def __init__(self):
ShowBase.__init__(self)
self.dialog = "Hello, how are you?"
self.options = ["Good", "Bad"]
self.dialogSystem = DialogSystem(self.dialog, self.options, self.onDialogSelect)
self.accept("space", self.showDialog)
def showDialog(self):
self.dialogSystem.show()
def onDialogSelect(self, value):
print("Selected option:", value)
app = MyApp()
app.run()
```
在这个示例中,我们创建了一个名为 `DialogSystem` 的对话系统类,该类包含对话内容、对话选项和回调函数。我们还创建了一个名为 `MyApp` 的游戏类,该类包含一个对话系统实例和一个显示对话框的方法。当用户选择对话选项时,我们会调用回调函数来处理用户的选择。
用panda3d写一个对话系统。要求1.能从文件中读取内容;2.能通过鼠标点击读取下一行。给出代码。
### 回答1:
好的,我可以帮助您用Panda3D编写一个对话系统,满足以下两个要求:
1. 能够从文件中读取对话内容。
2. 能够通过鼠标点击读取下一行对话。
下面是一个示例代码,可以实现这个功能:
```python
from direct.showbase.ShowBase import ShowBase
from direct.gui.DirectGui import *
import os
class DialogSystem(ShowBase):
def __init__(self):
ShowBase.__init__(self)
# 创建文本显示框
self.dialogBox = DirectFrame(
frameColor=(0, 0, 0, 0.6),
frameSize=(-1, 1, -0.3, 0.3),
pos=(0, 0, 0.7),
)
self.dialogText = OnscreenText(
text="",
pos=(0, 0),
scale=0.1,
fg=(1, 1, 1, 1),
align=TextNode.ACenter,
mayChange=True,
parent=self.dialogBox
)
# 创建按钮
self.nextButton = DirectButton(
text="Next",
scale=0.1,
pos=(0, 0, -0.3),
command=self.getNextLine,
parent=self.dialogBox
)
# 读取对话文件
self.dialogLines = []
with open("dialog.txt", "r", encoding="utf-8") as f:
for line in f:
self.dialogLines.append(line.strip())
# 初始化对话索引
self.dialogIndex = 0
# 显示第一行对话
self.dialogText.setText(self.dialogLines[self.dialogIndex])
def getNextLine(self):
# 增加对话索引
self.dialogIndex += 1
# 如果对话已经结束,关闭对话框
if self.dialogIndex >= len(self.dialogLines):
self.dialogBox.hide()
return
# 显示下一行对话
self.dialogText.setText(self.dialogLines[self.dialogIndex])
app = DialogSystem()
app.run()
```
这个代码假设您已经有了一个名为`dialog.txt`的对话文件,其中每一行代表一条对话内容。当程序运行时,会自动读取这个文件,并将其保存为一个列表。程序会在界面上显示一段对话内容和一个“Next”按钮。当用户点击这个按钮时,程序会显示下一行对话内容,直到对话结束。
### 回答2:
使用Panda3D编写一个对话系统,可以满足从文件中读取内容和通过鼠标点击读取下一行的要求。
下面是一个示例代码:
```python
from panda3d.core import TextNode, Vec3
from direct.showbase.ShowBase import ShowBase
class DialogSystem(ShowBase):
def __init__(self):
ShowBase.__init__(self)
# 创建一个文本节点用于显示对话内容
self.dialog_text = self.create_text_node()
# 加载对话内容文件
self.dialog_lines = self.load_dialog_lines("dialog.txt")
# 初始化当前对话行数
self.current_line = 0
# 添加鼠标点击事件
self.accept("mouse1", self.next_line)
# 初始显示第一行对话
self.show_line()
def create_text_node(self):
text_node = TextNode("dialog_text_node")
text_node.setTextColor(1, 1, 1, 1) # 设置字体颜色为白色
text_node.setAlign(TextNode.ACenter) # 设置文本对齐方式为居中
text_node.setCardColor(0, 0, 0, 0.6) # 设置文本背景色为半透明黑色
text_node_path = aspect2d.attachNewNode(text_node)
text_node_path.setScale(0.08) # 设置文本缩放大小
text_node_path.setPos(0, 0, -0.8) # 设置文本的位置
return text_node_path
def load_dialog_lines(self, file_path):
dialog_lines = []
with open(file_path, "r") as file:
for line in file:
dialog_lines.append(line.strip())
return dialog_lines
def show_line(self):
if self.current_line < len(self.dialog_lines):
line_text = self.dialog_lines[self.current_line]
self.dialog_text.node().setText(line_text)
self.current_line += 1
else:
self.dialog_text.node().setText("对话结束")
def next_line(self):
if self.current_line < len(self.dialog_lines):
self.show_line()
dialog_system = DialogSystem()
dialog_system.run()
```
上述代码创建了一个`DialogSystem`类,继承自`ShowBase`,用于创建一个Panda3D窗口并实现对话系统。在初始化函数`__init__`中,创建了一个文本节点用于显示对话内容,加载对话内容文件,并初始化当前对话行数为0。接着,添加了鼠标点击事件,定义了`next_line`函数和`show_line`函数。
`next_line`函数用于读取下一行对话,通过判断当前行数是否小于对话行数来确定是否还有下一行对话。`show_line`函数使用当前行数获取对应的对话内容,并在文本节点上显示出来。
最后,创建了一个`DialogSystem`对象,并调用其`run`函数启动Panda3D窗口运行对话系统。
请注意,以上代码仅仅是一个简单示例,实际使用中需要根据具体需求进行调整和扩展。
### 回答3:
下面是一个用Panda3D编写的基本对话系统的示例代码:
import direct.directbase.DirectStart
from direct.gui.OnscreenText import OnscreenText
from direct.showbase.DirectObject import DirectObject
class DialogSystem(DirectObject):
def __init__(self):
self.dialogLines = [] # 对话内容存储列表
self.currentLine = 0 # 当前正在显示的对话行索引
self.dialogBox = OnscreenText(text='', pos=(-1.3, 0.9), scale=0.07)
# 注册鼠标点击事件
self.accept('mouse1', self.nextLine)
def loadDialog(self, filename):
with open(filename, 'r') as file:
self.dialogLines = file.readlines()
def nextLine(self):
if self.currentLine < len(self.dialogLines):
self.dialogBox.setText(self.dialogLines[self.currentLine].strip())
self.currentLine += 1
dialogSys = DialogSystem()
dialogSys.loadDialog('dialog.txt')
dialogSys.nextLine()
run()
阅读全文
相关推荐
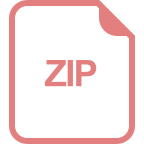
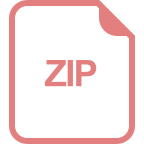
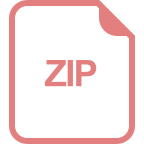








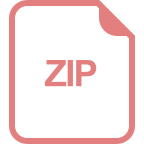
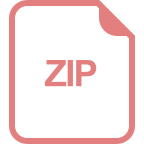
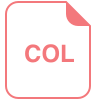
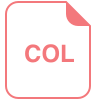
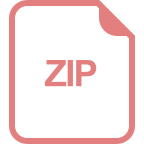
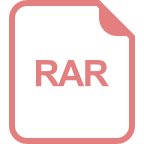