创建一个5行4列的矩阵m1,然后创建一个5行4列的矩阵m2,按行填充,再创建一个5行4列的矩阵m3,按列填充,再创建一个5行4列的矩阵m4,标注列名,最后取矩阵m1中第2行的所有元素 和矩阵m1中第2行,第3列的元素输出
时间: 2024-11-22 17:39:27 浏览: 16
在Python的NumPy库中,可以按照描述来创建和操作矩阵。首先,我们需要导入`numpy`模块,并创建所需的矩阵。
```python
import numpy as np
# 创建矩阵m1,所有元素默认为0
m1 = np.zeros((5, 4))
# 按行填充矩阵m2,这里假设有一个列表或数组values
row_values = [1, 2, 3, 4, 5] * 4 # 示例,每行都是这5个数
m2 = np.array(row_values).reshape(5, 4)
# 按列填充矩阵m3,同样需要预先准备数据
col_values = list(range(1, 5)) * 4
m3 = np.column_stack(col_values)
# 创建带列名的矩阵m4,这里我们只支持数值,列名通常是字符串,但你可以使用字典映射
col_names = ['A', 'B', 'C', 'D']
m4 = np.zeros((5, 4), dtype=object) # 使用object类型存储列名
m4[:, 0] = col_names
# 取矩阵m1的第2行
row_2_m1 = m1[1]
# 取矩阵m1的第2行,第3列的元素
element_2_3_m1 = row_2_m1[2]
print("Matrix m1 (2nd row):", row_2_m1)
print("Element at (2, 3) in matrix m1:", element_2_3_m1)
```
相关问题
R是m1×n1的二维表(整数矩阵)和S是m2×n2的二维表(整数矩阵),做大于联接运算。输入第一个表R的列序号a1和第二个表S的列序号a2。输出大于联接运算结果表C。以文件abc.out输出。
在关系数据库或数据处理领域,"大于连接(Greater Join)"通常指的是基于特定列值进行比较的自连接操作,而不是标准的SQL中的JOIN类型,比如INNER JOIN、LEFT JOIN等。在这个场景下,如果你有两个矩阵R和S,每个矩阵都是由整数组成的二维数组,你需要根据给定的列索引`a1`和`a2`,找出满足条件的行,即R的第`a1`列元素大于S的第`a2`列元素。
这是一个Python示例,假设我们有一个简单的函数来进行这个操作:
```cpp
#include <iostream>
#include <vector>
// 假设矩阵R和S存储在一个二维整数向量中
std::vector<std::vector<int>> R, S;
void greater_join(int a1, int a2) {
std::vector<std::pair<int, int>> result; // 存储结果的对(a1,R[i][a1],a2,S[j][a2])
for (const auto& row : R) {
for (const auto& col : S) {
if (row[a1] > col[a2]) { // 判断条件
result.push_back({a1, row[a1], a2, col[a2]}); // 添加到结果
}
}
}
// 写入到文件abc.out
std::ofstream file("abc.out");
for (const auto& pair : result) {
file << "Column " << pair.first << " in R: " << pair.second
<< ", Column " << pair.third << " in S: " << pair.fourth << "\n";
}
file.close();
}
int main() {
// 填充R和S的实际值
// ...
// 执行大于连接
int a1, a2;
// 获取用户输入的列索引
// ...
greater_join(a1, a2);
return 0;
}
```
这个代码首先定义了一个`greater_join`函数,用于执行实际的操作。在`main`函数里,你需要获取用户输入的`a1`和`a2`列索引,并确保这两个矩阵的尺寸兼容以便进行比较。
c++创建两个二维整数矩阵, 要求输出矩阵, 并计算两个矩阵的加法、乘法结果及其计算时间。尽量简便
在C++中,你可以使用`std::vector`来创建二维整数矩阵,并利用`chrono`库来测量时间和进行矩阵运算。下面是一个简单的示例,展示了如何创建矩阵、输出矩阵、以及实现矩阵的加法和乘法:
```cpp
#include <iostream>
#include <vector>
#include <chrono>
// 定义矩阵类
class Matrix {
private:
std::vector<std::vector<int>> data;
int rows, cols;
public:
// 构造函数
Matrix(int r, int c) : rows(r), cols(c) { data.resize(rows, std::vector<int>(cols, 0)); }
// 输出矩阵
void display() const {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j)
std::cout << data[i][j] << " ";
std::cout << "\n";
}
}
// 加法
Matrix operator+(const Matrix& other) {
if (rows != other.rows || cols != other.cols) throw std::runtime_error("Matrices must have the same dimensions for addition");
Matrix result(rows, cols);
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j)
result.data[i][j] = data[i][j] + other.data[i][j];
}
return result;
}
// 乘法(假设是元素级乘法)
Matrix operator*(const Matrix& other) {
if (cols != other.rows) throw std::runtime_error("Incompatible matrix dimensions for multiplication");
Matrix result(rows, other.cols);
auto start = std::chrono::high_resolution_clock::now();
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < other.cols; ++j) {
int sum = 0;
for (int k = 0; k < cols; ++k)
sum += data[i][k] * other.data[k][j];
result.data[i][j] = sum;
}
}
auto end = std::chrono::high_resolution_clock::now();
double time_spent = std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() / 1e6; // 转换为毫秒
std::cout << "Time taken for multiplication: " << time_spent << " ms\n";
return result;
}
};
int main() {
int r, c;
std::cout << "Enter number of rows and columns for each matrix: ";
std::cin >> r >> c;
Matrix m1(r, c), m2(r, c);
// 获取用户输入并填充矩阵
std::cout << "Enter elements for matrix 1:\n";
for (auto &row : m1.data)
for (auto &element : row)
std::cin >> element;
std::cout << "Enter elements for matrix 2:\n";
for (auto &row : m2.data)
for (auto &element : row)
std::cin >> element;
// 输出和运算
std::cout << "Matrix 1:\n" << m1 << "\n";
std::cout << "Matrix 2:\n" << m2 << "\n";
m1.display(); // 输出第一个矩阵
m2.display(); // 输出第二个矩阵
Matrix sum = m1 + m2;
std::cout << "Sum:\n" << sum << "\n";
Matrix product = m1 * m2;
std::cout << "Product:\n" << product << "\n";
return 0;
}
```
阅读全文
相关推荐

















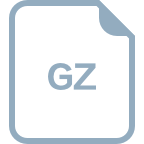