写一个python坦克大战小游戏
时间: 2024-05-29 09:03:42 浏览: 165
好的,下面是一个简单的Python坦克大战小游戏代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 创建游戏窗口
window = pygame.display.set_mode((800, 600))
pygame.display.set_caption("坦克大战")
# 加载游戏素材
tank_img = pygame.image.load("tank.png")
bullet_img = pygame.image.load("bullet.png")
enemy_tank_img = pygame.image.load("enemy_tank.png")
explosion_img = pygame.image.load("explosion.png")
# 设置游戏字体
font = pygame.font.SysFont(None, 30)
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
# 定义游戏对象类
class GameObject:
def __init__(self, x, y, img):
self.x = x
self.y = y
self.img = img
self.rect = self.img.get_rect()
self.rect.center = (self.x, self.y)
def draw(self, surface):
surface.blit(self.img, self.rect)
class Tank(GameObject):
def __init__(self, x, y, img):
super().__init__(x, y, img)
self.speed = 5
self.direction = "up"
def move(self):
if self.direction == "up":
self.y -= self.speed
elif self.direction == "down":
self.y += self.speed
elif self.direction == "left":
self.x -= self.speed
elif self.direction == "right":
self.x += self.speed
# 限制坦克移动范围
if self.x < 0:
self.x = 0
elif self.x > 800:
self.x = 800
if self.y < 0:
self.y = 0
elif self.y > 600:
self.y = 600
self.rect.center = (self.x, self.y)
def fire(self):
bullet = Bullet(self.x, self.y, self.direction)
return bullet
class Bullet(GameObject):
def __init__(self, x, y, direction):
self.speed = 10
self.direction = direction
if self.direction == "up":
img = pygame.transform.rotate(bullet_img, 90)
elif self.direction == "down":
img = pygame.transform.rotate(bullet_img, -90)
elif self.direction == "left":
img = pygame.transform.rotate(bullet_img, 180)
elif self.direction == "right":
img = bullet_img
super().__init__(x, y, img)
def move(self):
if self.direction == "up":
self.y -= self.speed
elif self.direction == "down":
self.y += self.speed
elif self.direction == "left":
self.x -= self.speed
elif self.direction == "right":
self.x += self.speed
self.rect.center = (self.x, self.y)
class EnemyTank(Tank):
def __init__(self, x, y, img):
super().__init__(x, y, img)
self.speed = 3
self.direction = random.choice(["up", "down", "left", "right"])
def update(self):
# 随机改变方向
if random.randint(0, 100) == 0:
self.direction = random.choice(["up", "down", "left", "right"])
self.move()
# 定义游戏函数
def game():
# 创建玩家坦克
player_tank = Tank(400, 500, tank_img)
# 创建敌方坦克
enemy_tank = EnemyTank(400, 100, enemy_tank_img)
enemy_tanks = [enemy_tank]
# 创建子弹列表
bullets = []
# 定义游戏循环
running = True
while running:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
player_tank.direction = "up"
elif event.key == pygame.K_DOWN:
player_tank.direction = "down"
elif event.key == pygame.K_LEFT:
player_tank.direction = "left"
elif event.key == pygame.K_RIGHT:
player_tank.direction = "right"
elif event.key == pygame.K_SPACE:
bullet = player_tank.fire()
bullets.append(bullet)
# 移动玩家坦克
player_tank.move()
# 移动敌方坦克
for enemy_tank in enemy_tanks:
enemy_tank.update()
# 移动子弹
for bullet in bullets:
bullet.move()
# 检测子弹是否击中敌方坦克
for bullet in bullets:
for enemy_tank in enemy_tanks:
if bullet.rect.colliderect(enemy_tank.rect):
bullets.remove(bullet)
enemy_tanks.remove(enemy_tank)
explosion = GameObject(enemy_tank.x, enemy_tank.y, explosion_img)
explosions.append(explosion)
# 绘制游戏对象
window.fill(black)
player_tank.draw(window)
for enemy_tank in enemy_tanks:
enemy_tank.draw(window)
for bullet in bullets:
bullet.draw(window)
for explosion in explosions:
explosion.draw(window)
# 更新游戏界面
pygame.display.update()
# 退出Pygame
pygame.quit()
# 运行游戏函数
game()
```
这只是一个简单的坦克大战小游戏,你可以根据自己的需要进行修改和扩展。
阅读全文
相关推荐
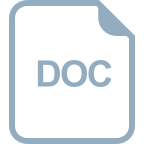
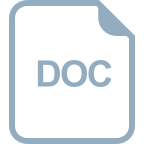
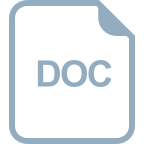


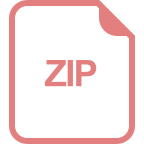
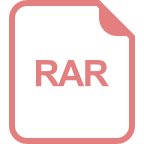
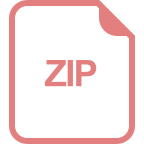
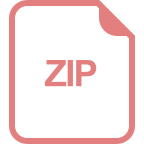









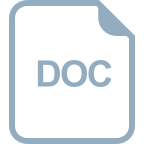