使用opencv和dlib库从摄像头中取人脸进行人眼检测的c++程序
时间: 2023-08-31 07:35:07 浏览: 70
### 回答1:
下面是一个使用 OpenCV 和 Dlib 库从摄像头中取人脸进行人眼检测的 C++ 程序示例:
```C++
#include <opencv2/opencv.hpp>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
using namespace cv;
using namespace dlib;
int main()
{
// 打开摄像头
VideoCapture capture(0);
if (!capture.isOpened())
{
std::cout << "摄像头打开失败" << std::endl;
return -1;
}
// 创建 Dlib 人脸检测器
frontal_face_detector detector = get_frontal_face_detector();
// 加载 Dlib 人脸关键点检测器
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
// 循环读取摄像头帧
Mat frame;
while (capture.read(frame))
{
// 将 OpenCV 图像转换为 Dlib 图像
cv_image<bgr_pixel> cimg(frame);
// 使用 Dlib 检测人脸
std::vector<rectangle> faces = detector(cimg);
if (faces.size() == 0)
{
imshow("人眼检测", frame);
waitKey(10);
continue;
}
// 循环遍历每个检测到的人脸
for (size_t i = 0; i < faces.size(); i++)
{
// 使用 Dlib 检测人脸关键点
full_object_detection shape = sp(cimg, faces[i]);
// 循环遍历每个关键点
for (size_t j = 0; j < shape.num_parts(); j++)
{
// 如果是眼睛关键点
if (j >= 36 && j <= 41)
{
// 在 OpenCV 图像中画出关键点
Point p(shape.part(j).x(), shape.part(j).y());
circle(frame, p, 2, Scalar(0, 255, 0), -1);
}
}
}
// 显示
### 回答2:
使用opencv和dlib库从摄像头中提取人脸并进行眼部检测,可以通过以下步骤实现:
1. 导入所需库文件,包括opencv和dlib。
```c++
#include <opencv2/opencv.hpp>
#include <dlib/opencv.h>
#include <dlib/image_processing.h>
```
2. 创建一个视频捕捉对象,用于连接电脑上的摄像头。
```c++
cv::VideoCapture capture(0); // 参数0表示连接默认摄像头
```
3. 加载dlib人脸检测器和眼部检测器。
```c++
dlib::frontal_face_detector detector = dlib::get_frontal_face_detector();
dlib::shape_predictor predictor;
```
4. 加载用于检测人眼的dlib预训练模型。
```c++
dlib::deserialize("shape_predictor_68_face_landmarks.dat") >> predictor;
```
5. 通过循环和帧处理每一帧图像,进行人脸和眼部检测。
```c++
while (true) {
cv::Mat frame;
capture >> frame; // 读取一帧图像
cv::Mat gray_frame;
cv::cvtColor(frame, gray_frame, cv::COLOR_BGR2GRAY); // 转换为灰度图像
// 使用dlib检测人脸
std::vector<dlib::rectangle> faces = detector(dlib::cv_image<unsigned char>(gray_frame));
for (int i = 0; i < faces.size(); i++) {
dlib::rectangle face = faces[i];
// 使用dlib检测眼部
dlib::full_object_detection shape = predictor(dlib::cv_image<unsigned char>(gray_frame), face);
// 绘制眼部边界框
for (int j = 36; j <= 47; j++) {
cv::Point eye(shape.part(j).x(), shape.part(j).y());
cv::circle(frame, eye, 2, cv::Scalar(0, 0, 255), -1);
}
}
// 显示处理后的帧图像
cv::imshow("Face and Eye Detection", frame);
// 按下ESC键退出程序
if (cv::waitKey(1) == 27) break;
}
```
6. 释放相关资源并关闭窗口。
```c++
capture.release();
cv::destroyAllWindows();
```
通过以上步骤,我们就可以使用opencv和dlib库实现一个从摄像头中提取人脸并进行眼部检测的C程序。
相关推荐
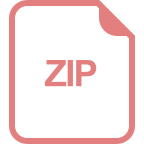
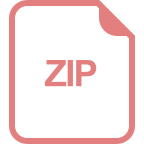
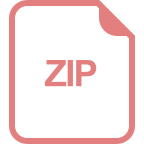
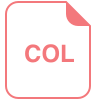
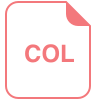
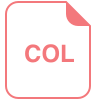
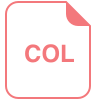
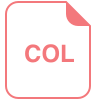









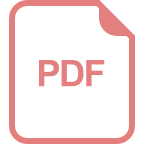