TypeError: randn(): argument 'size' (position 1) must be tuple of ints, not numpy.ndarray
时间: 2024-05-04 12:20:02 浏览: 9
这个错误通常是由于传递到 `randn()` 函数的参数类型不正确导致的。`randn()` 函数的第一个参数 `'size'` 应该是一个元组类型的整数,而不是一个 numpy 数组。
你需要检查传递给 `randn()` 函数的参数类型是否正确。如果你传递的是一个 numpy 数组,你需要将其转换为元组类型的整数。你可以使用 `tuple()` 函数将 numpy 数组转换为元组类型的整数,例如:
```python
import numpy as np
# 将 numpy 数组转换为元组类型的整数
size = tuple(np.array([2, 3]))
# 使用正确的参数调用 randn() 函数
x = np.random.randn(*size)
```
这将把 numpy 数组 `[2, 3]` 转换为元组 `(2, 3)`,然后使用正确的参数调用 `randn()` 函数。
相关问题
TypeError: randn(): argument 'size' must be tuple of ints, but found element of type Tensor at pos 2
这个错误通常是由于在使用PyTorch的`torch.randn()`函数时,将一个张量(Tensor)作为参数传递而不是一个整数元组。`torch.randn()`函数的参数应该是一个整数元组,用于指定返回张量的形状。例如,`torch.randn((3, 4))`将返回一个3x4的张量。
请检查你的代码,确认所有使用`torch.randn()`函数的地方都传递一个整数元组作为参数,而不是一个张量。
TypeError: linear(): argument 'input' (position 1) must be Tensor, not numpy.ndarray
这是一个 Python 的错误提示信息,它通常说明你试图使用一个numpy数组作为输入参数传递给 PyTorch中的线性层(linear layer)。然而,线性层要求输入参数必须是 PyTorch tensor 类型。要解决这个问题,你需要将 numpy 数组转化为 PyTorch tensor,可以使用 PyTorch 中的 from_numpy() 方法。代码示例如下:
```python
import torch
#从numpy数组中创建一个PyTorch tensor
input_np_array = np.ones((2,3))
input_tensor = torch.from_numpy(input_np_array)
#使用线性层来处理输入tensor
linear_layer = torch.nn.Linear(3,4)
output_tensor = linear_layer(input_tensor)
```
在这个例子中,我们首先使用numpy创建一个形状为(2,3)的数组。然后,我们使用 torch.from_numpy() 方法将它转换成一个 PyTorch tensor 对象。最后,我们使用 PyTorch 中的线性层来处理这个 tensor。这里我们的线性层输入的形状是 (3,4)。注意,这里我们并没有使用 numpy 数组或者将 numpy 数组直接传递给线性层作为输入,而是将 numpy 数组转换为了 PyTorch tensor,然后将这个 tensor 输入到线性层中。这样就可以避免上述的 TypeError 错误。
相关推荐
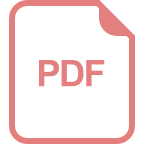
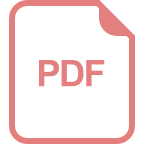
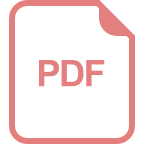












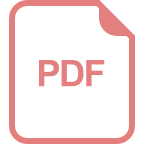