TypeError: linear(): argument 'input' (position 1) must be Tensor, not numpy.ndarray 如何解决
时间: 2024-03-20 08:39:32 浏览: 270
这个错误通常发生在你试图将一个 NumPy 数组(`numpy.ndarray`)传递给 PyTorch 神经网络的线性层(`torch.nn.Linear`)作为输入,而线性层要求的输入必须是 PyTorch 张量(`torch.Tensor`)。
要解决这个错误,你需要将 NumPy 数组转换为 PyTorch 张量。可以使用 `torch.from_numpy()` 方法将 NumPy 数组转换为 PyTorch 张量。例如:
```
import numpy as np
import torch
# 创建一个 2x3 的 NumPy 数组
arr = np.array([[1, 2, 3], [4, 5, 6]])
# 将 NumPy 数组转换为 PyTorch 张量
tensor = torch.from_numpy(arr)
# 创建一个线性层
linear = torch.nn.Linear(3, 2)
# 将张量作为输入传递给线性层
output = linear(tensor)
```
在这个例子中,我们首先创建一个 2x3 的 NumPy 数组 `arr`,然后使用 `torch.from_numpy()` 方法将其转换为 PyTorch 张量 `tensor`。接着,我们创建了一个输入大小为 3,输出大小为 2 的线性层 `linear`,然后将张量 `tensor` 作为输入传递给线性层,并将输出保存在 `output` 变量中。这样就可以避免上述错误了。
相关问题
TypeError: linear(): argument 'input' (position 1) must be Tensor, not numpy.ndarray
这是一个 Python 的错误提示信息,它通常说明你试图使用一个numpy数组作为输入参数传递给 PyTorch中的线性层(linear layer)。然而,线性层要求输入参数必须是 PyTorch tensor 类型。要解决这个问题,你需要将 numpy 数组转化为 PyTorch tensor,可以使用 PyTorch 中的 from_numpy() 方法。代码示例如下:
```python
import torch
#从numpy数组中创建一个PyTorch tensor
input_np_array = np.ones((2,3))
input_tensor = torch.from_numpy(input_np_array)
#使用线性层来处理输入tensor
linear_layer = torch.nn.Linear(3,4)
output_tensor = linear_layer(input_tensor)
```
在这个例子中,我们首先使用numpy创建一个形状为(2,3)的数组。然后,我们使用 torch.from_numpy() 方法将它转换成一个 PyTorch tensor 对象。最后,我们使用 PyTorch 中的线性层来处理这个 tensor。这里我们的线性层输入的形状是 (3,4)。注意,这里我们并没有使用 numpy 数组或者将 numpy 数组直接传递给线性层作为输入,而是将 numpy 数组转换为了 PyTorch tensor,然后将这个 tensor 输入到线性层中。这样就可以避免上述的 TypeError 错误。
TypeError: nll_loss_nd(): argument 'input' (position 1) must be Tensor, not numpy.ndarray
This error occurs when the input argument for the nll_loss_nd() function is a numpy array instead of a PyTorch tensor.
To fix this error, you can convert the numpy array to a PyTorch tensor using the from_numpy() method.
Here is an example code snippet:
```
import torch
import numpy as np
# create a numpy array
arr = np.array([1, 2, 3])
# convert the numpy array to a PyTorch tensor
tensor = torch.from_numpy(arr)
# use the tensor as input for nll_loss_nd() function
loss = torch.nn.functional.nll_loss_nd(tensor)
```
阅读全文
相关推荐
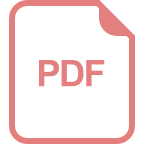
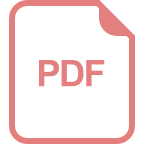
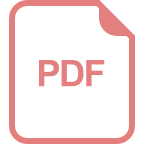













