在python用CFFI调用c的dll时 TypeError: initializer for ctype 'double *' must be a cdata pointer, not numpy.ndarray
时间: 2023-07-16 17:15:42 浏览: 107
这个错误通常是由于使用了numpy数组作为CFFI中的ctype类型时出现的。由于numpy数组不是C数据类型,因此需要将其转换为C数据类型才能在CFFI中使用。
要解决这个问题,需要使用CFFI提供的“from_buffer”方法将numpy数组转换为C数据类型。下面是一个示例代码:
```python
import numpy as np
from cffi import FFI
ffi = FFI()
# 定义C函数签名
ffi.cdef("void my_function(double *data, int size);")
# 加载C库
lib = ffi.dlopen("my_library.dll")
# 创建一个numpy数组
my_array = np.array([1.0, 2.0, 3.0])
# 将numpy数组转换为C数据类型
my_c_array = ffi.cast("double *", my_array.ctypes.data)
# 调用C函数
lib.my_function(my_c_array, len(my_array))
```
在上面的示例代码中,使用“ffi.cast”方法将numpy数组转换为C数据类型。这里假设“my_function”函数需要一个双精度浮点数指针作为参数。
相关问题
TypeError: Value after * must be an iterable, not numpy.uint8
This error occurs when you try to multiply a single value (which is not iterable) with a numpy array. To fix this error, you need to ensure that both operands of the multiplication operation are iterable.
For example, if you have a numpy array `a` and a scalar value `b`, you can multiply them using the following code:
```
import numpy as np
a = np.array([1, 2, 3])
b = 2
result = a * b
```
In this example, `b` is a scalar value, but it is automatically broadcasted to match the shape of `a` (which is `[1, 2, 3]`). This means that `b` is effectively converted to the array `[2, 2, 2]`, and then multiplied element-wise with `a` to produce the result `[2, 4, 6]`.
If you still get the same error, make sure that the data type of your numpy array is compatible with the scalar value you want to use for multiplication. For example, if your numpy array has a data type of `uint8`, you should use a scalar value that can be converted to `uint8`, such as an integer between 0 and 255.
TypeError: order must be str, not numpy.ndarray
`: order must be str, not numpy.ndarray` 错误表示传递给排序函数的 `order` 参数必须是字符串,而不是 NumPy 数组。
在 Python 中,如果使用内置的 `sorted()` 函数进行排序,可以将排序关键字传递为字符串。例如,如果要按照字符串长度对列表进行排序,可以传递 `key=len`。
如果使用 NumPy 库的排序函数(如 `np.sort()` 或 `ndarray.sort()`),则需要将排序关键字作为 `order` 参数传递。该参数必须是字符串类型,用于指定要排序的字段。例如,如果要按照第一列排序多维数组,可以使用 `order='f0'`。
以下是一个示例代码,演示了如何在 NumPy 中使用 `order` 参数进行排序:
```python
import numpy as np
# 创建一个二维数组
a = np.array([(1, 3), (2, 1), (3, 2)], dtype=[('x', int), ('y', int)])
print('Original array:')
print(a)
# 按照第一列排序
b = np.sort(a, order='x')
print('Sorted array by x:')
print(b)
# 按照第二列排序
c = np.sort(a, order='y')
print('Sorted array by y:')
print(c)
```
在上面的示例中,我们首先创建了一个二维数组 `a`,该数组包含两个字段 `x` 和 `y`,分别表示第一列和第二列。然后,我们使用 `np.sort()` 方法按照第一列和第二列分别对数组进行排序,并将结果保存在 `b` 和 `c` 变量中。最后,我们打印排序后的数组。
需要注意的是,在使用 `order` 参数进行排序时,必须确保字段名称与数组中的字段名称相匹配,否则会出现 `KeyError` 错误。
阅读全文
相关推荐
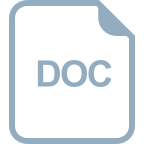
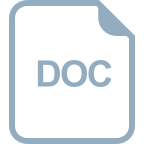
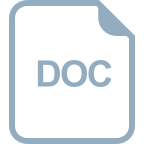













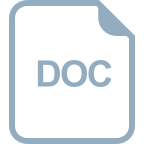