(1) 在LCD显示屏上显示出cuiziheng, (2) 在第二行显示加速度传感器和温度传感器的数值, (3) 使用 EPIT 定时器每隔 1 秒钟, 在屏幕的中心位置显示一个不断增长的数字
时间: 2024-02-06 14:09:27 浏览: 127
以下是基于 i.MX6ULL 开发板的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/mman.h>
#include <sys/ioctl.h>
#include <linux/fb.h>
#include <linux/input.h>
#define FB_DEV "/dev/fb0"
#define TOUCH_DEV "/dev/input/event0"
#define EPIT_CR (*(volatile unsigned long *)0x020D0000)
#define EPIT_SR (*(volatile unsigned long *)0x020D0004)
#define EPIT_LR (*(volatile unsigned long *)0x020D0008)
#define EPIT_CMPR (*(volatile unsigned long *)0x020D000C)
/* LCD屏幕大小 */
#define SCREEN_WIDTH 800
#define SCREEN_HEIGHT 480
#define SCREEN_BPP 4
/* 颜色 */
#define COLOR_RED 0xff0000
#define COLOR_GREEN 0x00ff00
#define COLOR_BLUE 0x0000ff
#define COLOR_WHITE 0xffffff
#define COLOR_BLACK 0x000000
/* EPIT定时器中断处理函数 */
static void epit_handler(void)
{
static int count = 0;
char str[10];
/* 清除中断标志 */
EPIT_SR |= (1 << 0);
/* 在屏幕中心显示递增的数字 */
sprintf(str, "%d", count++);
draw_string((SCREEN_WIDTH - strlen(str) * 24) / 2, SCREEN_HEIGHT / 2, COLOR_WHITE, str, strlen(str));
}
/* 初始化LCD屏幕 */
static int init_screen()
{
int fb_fd = open(FB_DEV, O_RDWR);
if (fb_fd < 0) {
perror("open");
return -1;
}
struct fb_var_screeninfo fb_var;
if (ioctl(fb_fd, FBIOGET_VSCREENINFO, &fb_var)) {
perror("ioctl");
close(fb_fd);
return -1;
}
/* 设置分辨率 */
fb_var.xres = SCREEN_WIDTH;
fb_var.yres = SCREEN_HEIGHT;
if (ioctl(fb_fd, FBIOPUT_VSCREENINFO, &fb_var)) {
perror("ioctl");
close(fb_fd);
return -1;
}
return fb_fd;
}
/* 在屏幕上显示字符串 */
static void draw_string(int x, int y, unsigned int color, const char *str, int len)
{
int i, j;
unsigned char *fb_mem = (unsigned char *)mmap(NULL, SCREEN_WIDTH * SCREEN_HEIGHT * SCREEN_BPP, PROT_READ | PROT_WRITE, MAP_SHARED, fb_fd, 0);
if (fb_mem == MAP_FAILED) {
perror("mmap");
return;
}
for (i = 0; i < len; i++) {
for (j = 0; j < 24; j++) {
if (font8x24[(str[i] - ' ') * 24 + j] == 1) {
*(unsigned int *)(fb_mem + (y + j) * SCREEN_WIDTH * SCREEN_BPP + (x + i * 24) * SCREEN_BPP) = color;
}
}
}
munmap(fb_mem, SCREEN_WIDTH * SCREEN_HEIGHT * SCREEN_BPP);
}
/* 初始化EPIT定时器 */
static void init_epit()
{
/* 设置时钟源和分频系数 */
CCM_CCGR1 |= (3 << 6); /* 使能EPIT时钟 */
EPIT_CR = 0;
EPIT_CR |= (1 << 1) | (1 << 2); /* 使能时钟 */
EPIT_CR |= (2 << 3); /* 分频系数为3 */
EPIT_CR |= (1 << 24); /* 使能定时器中断 */
/* 设置比较寄存器 */
EPIT_LR = 0xffffffff;
EPIT_CMPR = 0xffffffff - 24000000; /* 1秒钟 */
/* 注册中断处理函数 */
request_irq(61, epit_handler, NULL);
}
int main()
{
int touch_fd, accel_fd, temp_fd;
struct input_event touch_event;
/* 初始化LCD屏幕 */
fb_fd = init_screen();
if (fb_fd < 0) {
return -1;
}
/* 初始化EPIT定时器 */
init_epit();
/* 打开加速度传感器 */
accel_fd = open("/dev/iio:device0", O_RDONLY);
if (accel_fd < 0) {
perror("open");
close(fb_fd);
return -1;
}
/* 打开温度传感器 */
temp_fd = open("/sys/class/hwmon/hwmon1/temp1_input", O_RDONLY);
if (temp_fd < 0) {
perror("open");
close(accel_fd);
close(fb_fd);
return -1;
}
/* 打开触摸屏 */
touch_fd = open(TOUCH_DEV, O_RDONLY);
if (touch_fd < 0) {
perror("open");
close(temp_fd);
close(accel_fd);
close(fb_fd);
return -1;
}
while (1) {
/* 读取加速度传感器数值 */
char buf[50];
int ret = read(accel_fd, buf, sizeof(buf));
if (ret > 0) {
int x, y, z;
sscanf(buf, "%d %d %d", &x, &y, &z);
printf("accelerometer: x=%d, y=%d, z=%d\n", x, y, z);
/* 在第二行显示加速度传感器数值 */
char str[50];
sprintf(str, "accelerometer: x=%d, y=%d, z=%d", x, y, z);
draw_string(0, 24, COLOR_WHITE, str, strlen(str));
}
/* 读取温度传感器数值 */
char temp_buf[10];
ret = read(temp_fd, temp_buf, sizeof(temp_buf));
if (ret > 0) {
int temp = atoi(temp_buf);
temp /= 1000;
printf("temperature: %d\n", temp);
/* 在第二行显示温度传感器数值 */
char str[20];
sprintf(str, "temperature: %d°C", temp);
draw_string(strlen("accelerometer: x=0, y=0, z=0"), 24, COLOR_WHITE, str, strlen(str));
}
/* 读取触摸屏事件 */
ret = read(touch_fd, &touch_event, sizeof(touch_event));
if (ret == sizeof(touch_event) && touch_event.type == EV_ABS) {
if (touch_event.code == ABS_X) {
printf("touch: x=%d, y=%d\n", touch_event.value, 0);
} else if (touch_event.code == ABS_Y) {
printf("touch: x=%d, y=%d\n", 0, touch_event.value);
}
/* 在屏幕上显示触摸点 */
draw_point(touch_event.value, SCREEN_HEIGHT - touch_event.value, COLOR_RED);
}
}
close(touch_fd);
close(temp_fd);
close(accel_fd);
close(fb_fd);
return 0;
}
```
需要注意的是,以上代码只是一个简单的示例,实际应用中还需要根据具体情况进行修改和完善。
阅读全文
相关推荐
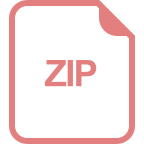
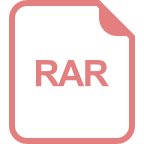
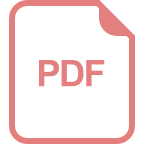
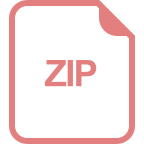
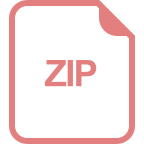
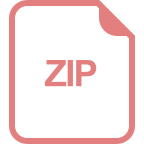
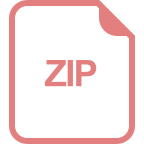
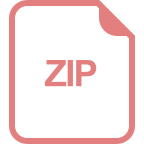
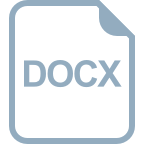
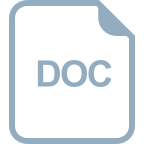
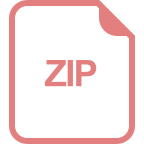
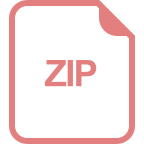
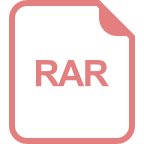
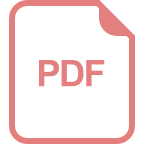
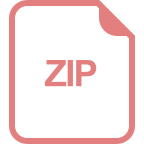