python计算cosx
时间: 2023-07-08 16:54:12 浏览: 234
要计算cos(x)函数,需要使用Python内置的数学库`math`。具体步骤如下:
1. 首先导入`math`库
```python
import math
```
2. 调用`math`库中的`cos()`函数,传入需要计算的角度参数,单位是弧度。
```python
cos_value = math.cos(x)
```
其中`x`是角度值,需要将其转换为弧度后传入`cos()`函数,可以使用`math.radians()`函数将角度转换为弧度。例如,计算`cos(30°)`的值,可以这样写:
```python
import math
x = math.radians(30) # 将角度转换为弧度
cos_value = math.cos(x)
print(cos_value)
```
输出结果为:
```
0.8660254037844387
```
这是`cos(30°)`的值,保留了小数点后15位。
相关问题
基于python在cosx折线图上填充|x|<0.5或cosx>0.5的区域为绿色,透明度为0.25
在Python中,你可以使用matplotlib库创建cosine曲线,并通过plt.fill_between函数来填充特定条件下的区域。为了基于`x`轴值的绝对值小于0.5或`cos(x)`大于0.5的情况填充绿色,你可以按照下面的步骤操作:
首先,确保已经安装了`matplotlib`库。如果没有,可以使用pip安装:
```bash
pip install matplotlib
```
然后,使用以下代码作为示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义x范围和计算cos(x)
x = np.linspace(-np.pi, np.pi, 400) # 创建400个等间距点从-pi到pi
y = np.cos(x)
# 创建一个新的图形
fig, ax = plt.subplots()
# 使用plt.fill_between绘制区域
ax.fill_between(x, y, where=(np.abs(x) < 0.5) | (y > 0.5), color='green', alpha=0.25)
# 设置轴标签和标题
ax.set_xlabel('X')
ax.set_ylabel('Cos(X)')
ax.set_title('Filled Regions for |x| < 0.5 and cos(x) > 0.5')
# 显示图形
plt.show()
```
这个代码会生成一个cosine曲线,绿色部分代表满足`|x| < 0.5`或`cos(x) > 0.5`的区域,填充颜色为绿色,透明度设为0.25。
如果你有其他关于如何修改图形、添加更多自定义或其他相关问题,请告诉我。
Python程序实现cosx
在Python中,你可以使用内置的数学库`math`来计算余弦值(cos)。这里是一个简单的例子:
```python
import math
def cosx(x):
"""
计算给定角度x的余弦值。
:param x: 角度,以弧度为单位
:return: x的余弦值
"""
return math.cos(x)
# 示例
angle_in_degrees = 45 # 也可以用弧度,例如 angle_in_radians = math.radians(45)
cos_value = cosx(math.radians(angle_in_degrees)) # 使用弧度值
print(f"cos({angle_in_degrees}°) = {cos_value}")
```
如果你想要使用角度而不是弧度,记得先将角度转换成弧度,因为`math.cos`函数默认接受弧度作为参数。
阅读全文
相关推荐
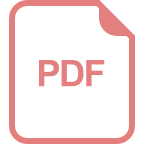













