java 删除给定链表中的重复元素(链表中元素从小到大有序排列),使链表中的所有元素都只出现一次 一行内输入一串整数,以空格间隔,以# 结束 一串去重后整数,以空格间隔,以# 结束。
时间: 2024-04-08 14:34:59 浏览: 85
您可以使用Java中的链表来实现删除给定链表中的重复元素。以下是一个示例代码:
```java
import java.util.*;
class ListNode {
int val;
ListNode next;
ListNode(int val) {
this.val = val;
this.next = null;
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String input = scanner.nextLine();
String[] numbers = input.split(" ");
ListNode head = new ListNode(Integer.parseInt(numbers[0]));
ListNode current = head;
for (int i = 1; i < numbers.length; i++) {
int num = Integer.parseInt(numbers[i]);
current.next = new ListNode(num);
current = current.next;
}
ListNode uniqueList = removeDuplicates(head);
StringBuilder result = new StringBuilder();
while (uniqueList != null) {
result.append(uniqueList.val).append(" ");
uniqueList = uniqueList.next;
}
System.out.println(result.toString().trim());
}
private static ListNode removeDuplicates(ListNode head) {
if (head == null) {
return null;
}
ListNode current = head;
while (current != null && current.next != null) {
if (current.val == current.next.val) {
current.next = current.next.next;
} else {
current = current.next;
}
}
return head;
}
}
```
在代码中,我们首先将输入的一行数字按空格分隔成一个字符串数组。然后,我们根据数字数组创建链表,并使用`removeDuplicates()`方法删除重复的元素。最后,我们将去重后的链表转换为字符串,并打印输出。
希望能帮到您!如果您还有其他问题,请随时提问。
阅读全文
相关推荐







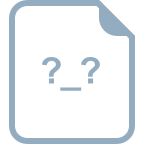
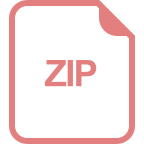
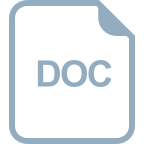
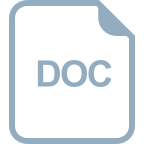



