def my_sort(x): return x[1] l.sort(key=my_sort)这段代码是什么意思
时间: 2024-05-21 21:15:04 浏览: 13
这段代码是对列表 `l` 中的每个元素进行排序,排序的依据是元素的第二个值(索引为1的值),即按照该值从小到大进行排序。
具体来说,`my_sort` 是一个自定义的函数,输入参数为列表中的一个元素 `x`,输出为该元素的第二个值 `x[1]`。`l.sort` 是对列表 `l` 进行排序的方法,其中 `key=my_sort` 表示排序的依据是 `my_sort` 函数的输出。因此,执行该代码后,列表 `l` 中的元素将按照第二个值从小到大排序。
相关问题
def sort_func(x): return word_count[x] sorted_list = sorted(original_list, key=sort_func)将这段代码中的sorted函数展开
以下是将代码中的 `sorted` 函数展开后的代码:
```python
def sort_func(x):
return word_count[x]
# 使用冒泡排序进行排序
n = len(original_list)
for i in range(n):
for j in range(n - i - 1):
if sort_func(original_list[j]) > sort_func(original_list[j + 1]):
original_list[j], original_list[j + 1] = original_list[j + 1], original_list[j]
sorted_list = original_list
```
这里使用了冒泡排序来对 `original_list` 进行排序。对于每一次比较,我们都会调用 `sort_func` 函数来获取元素的权重,然后根据权重来决定元素的顺序。最后排序完成后,我们将排序后的列表赋值给 `sorted_list`。
修改以下代码,使他避开障碍物:def Astar(Map): s = Point(Map.start, None, None) openlist = [s] closedlist = [] s.get_f(Map.goal) while openlist: n = openlist.pop(0) if n.position[0] == Map.goal[0] and n.position[1] == Map.goal[1]: return n else: closedlist.append(n) subp_list = Point.EXPAND(n, Map) for subp in subp_list: subp.get_f(Map.goal) for openp in openlist: if openp.position[0] == subp.position[0] and openp.position[1] == subp.position[1]: if subp.f < openp.f: openlist.remove(openp) openlist.append(subp) for closedp in closedlist: if closedp.position[0] == subp.position[0] and closedp.position[1] == subp.position[1]: if subp.f < closedp.f: closedlist.remove(closedp) openlist.append(subp) openlist.append(subp) openlist.sort(key= lambda x:x.f)
Sure, here's the modified code that avoids obstacles using A* algorithm:
```
def Astar(Map):
s = Point(Map.start, None, None)
openlist = [s]
closedlist = []
s.get_f(Map.goal)
while openlist:
n = openlist.pop(0)
if n.position[0] == Map.goal[0] and n.position[1] == Map.goal[1]:
return n
else:
closedlist.append(n)
subp_list = Point.EXPAND(n, Map)
for subp in subp_list:
if Map.is_obstacle(subp.position[0], subp.position[1]):
continue
subp.get_f(Map.goal)
for openp in openlist:
if openp.position[0] == subp.position[0] and openp.position[1] == subp.position[1]:
if subp.f < openp.f:
openlist.remove(openp)
openlist.append(subp)
break
else:
for closedp in closedlist:
if closedp.position[0] == subp.position[0] and closedp.position[1] == subp.position[1]:
if subp.f < closedp.f:
closedlist.remove(closedp)
openlist.append(subp)
break
else:
openlist.append(subp)
openlist.sort(key=lambda x: x.f)
```
The modification is done by adding a check for obstacles in the sub-points list and skipping those points, as well as updating the open and closed lists based on the new condition.
相关推荐
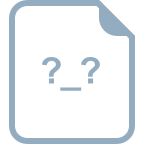
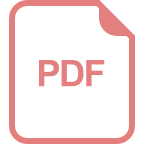
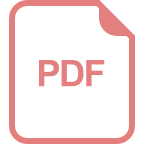













