c++利用c++利用基数排序对5000个随机数进行排序
时间: 2024-11-15 18:32:06 浏览: 1
基数排序是一种非比较整数排序算法,它通过将整数按位数切割成不同的数字,然后按每个位数分别排序,最后再组合起来得到有序序列。在C++中实现基数排序针对5000个随机数,一般步骤如下:
1. **确定最大数**:首先找到数组中的最大数,确定需要排序的最大位数。
2. **设置桶**:创建大小为10(因为我们通常用的是10进制,所以用10个桶代表每一位)的桶数组,用于存放相应位上数值的计数。
3. **填充桶**:从最低位开始,对每个数字进行遍历,统计当前位上各数字的数量并放入对应的桶中。
4. **累计计数**:对于每个桶,计算累积值并将结果存放在一个新的数组里,这个过程相当于把每个桶内的数移动到正确的位置。
5. **收集元素**:从最低位到最高位依次重复上述过程,每次处理的范围是当前位及其后的所有位。每轮结束后,将所有桶合并到原始数组中,形成新的有序部分。
6. **循环结束**:当处理完所有的位后,整个数组就会按照基数排序完成。
```cpp
#include <iostream>
#include <vector>
#include <cmath>
void countingSort(std::vector<int>& arr, int exp) {
int n = arr.size();
std::vector<int> output(n);
std::vector<int> count(10, 0); // 10 for radix (base)
// Count occurrences of each digit in current position
for (int i = 0; i < n; i++) {
count[(arr[i] / exp) % 10]++;
}
// Modify count array such that count[i] now contains actual position of this digit in output array
for (int i = 1; i < 10; i++)
count[i] += count[i - 1];
// Build the output array
for (int i = n - 1; i >= 0; i--) {
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
// Copy the sorted elements into original array
for (int i = 0; i < n; i++)
arr[i] = output[i];
}
// Radix sort function
void radixsort(std::vector<int>& arr) {
int maxVal = *std::max_element(arr.begin(), arr.end());
int exp = 1;
while (maxVal / exp > 0) {
countingSort(arr, exp);
exp *= 10;
}
}
int main() {
std::srand(time(0));
std::vector<int> randomNumbers(5000, 0);
// Generate 5000 random numbers here...
radixsort(randomNumbers);
for (const auto& num : randomNumbers)
std::cout << num << " ";
std::cout << "\n";
return 0;
}
```
阅读全文
相关推荐
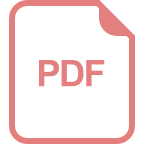
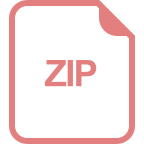
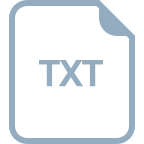
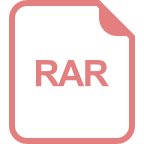
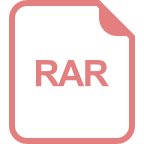
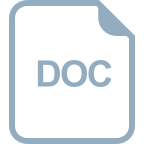
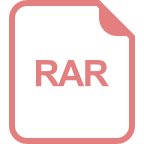
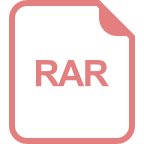
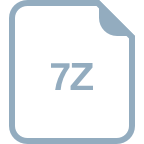
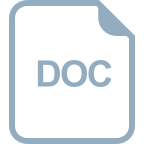
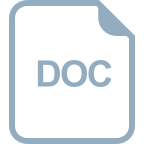
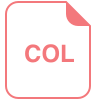





