C++ 判断一个5×5的二维数组是否对称?所谓对称是指所有x[i][j]元素与x[j][i]元素相等。
时间: 2023-09-22 09:15:27 浏览: 50
判断一个5×5的二维数组是否对称,可以按照如下步骤进行:
1. 遍历二维数组,判断x[i][j]是否等于x[j][i],如果不等于,则不是对称的,直接返回false。
2. 如果遍历完成,没有发现不相等的元素,则说明二维数组是对称的,返回true。
下面是实现代码:
```c++
#include <iostream>
using namespace std;
const int n = 5;
bool isSymmetric(int x[][n]) {
for(int i = 0; i < n; i++) {
for(int j = 0; j < n; j++) {
if(x[i][j] != x[j][i]) {
return false;
}
}
}
return true;
}
int main() {
int x[n][n] = {{1, 2, 3, 4, 5},
{2, 6, 7, 8, 9},
{3, 7, 10, 11, 12},
{4, 8, 11, 13, 14},
{5, 9, 12, 14, 15}};
if(isSymmetric(x)) {
cout << "The array is symmetric." << endl;
} else {
cout << "The array is not symmetric." << endl;
}
return 0;
}
```
上述代码中,首先定义了一个常量n为5,表示二维数组的大小为5×5。然后,isSymmetric函数遍历二维数组,判断是否对称,如果是对称的,则返回true,否则返回false。最后,在主函数中定义一个5×5的二维数组,并调用isSymmetric函数进行判断。如果二维数组是对称的,则输出"The array is symmetric.",否则输出"The array is not symmetric."。
相关推荐
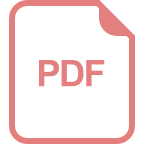
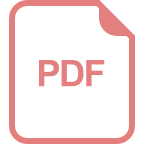














