(1)编写日期类,要求 a.用成员函数实现日期类 “<”运算符的重载。 b. 用友元函数实现日期类“=”运算符的重载 c.用成员函数实现日期类 “++”运算符的重载
时间: 2023-10-09 19:15:04 浏览: 107
好的,我来为您编写一个日期类的示例代码,满足您的要求:
```c++
#include <iostream>
using namespace std;
class Date {
private:
int year, month, day;
public:
Date(int y = 1970, int m = 1, int d = 1): year(y), month(m), day(d) { }
bool operator < (const Date& d) const {
if (year < d.year) {
return true;
} else if (year == d.year) {
if (month < d.month) {
return true;
} else if (month == d.month) {
return day < d.day;
}
}
return false;
}
Date& operator ++ () {
day++;
if (day > getDaysInMonth()) {
day = 1;
month++;
if (month > 12) {
month = 1;
year++;
}
}
return *this;
}
friend bool operator == (const Date& d1, const Date& d2) {
return d1.year == d2.year && d1.month == d2.month && d1.day == d2.day;
}
friend ostream& operator << (ostream& os, const Date& d) {
os << d.year << "年" << d.month << "月" << d.day << "日";
return os;
}
private:
int getDaysInMonth() const {
int daysInMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (month == 2 && isLeapYear()) {
return 29;
}
return daysInMonth[month-1];
}
bool isLeapYear() const {
return year % 400 == 0 || (year % 4 == 0 && year % 100 != 0);
}
};
int main() {
Date d1(2021, 7, 1);
Date d2(2022, 1, 1);
cout << (d1 < d2) << endl; // 输出 1
Date d3(2021, 7, 1);
Date d4(2021, 7, 1);
cout << (d3 == d4) << endl; // 输出 1
Date d5(2021, 6, 30);
++d5;
cout << d5 << endl; // 输出 2021年7月1日
return 0;
}
```
其中,重载运算符 `<` 和 `++` 是成员函数,重载运算符 `=` 是友元函数。此外,我们还重载了 `<<` 运算符,使其能够方便地输出日期对象的信息。
阅读全文
相关推荐



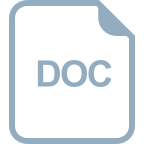
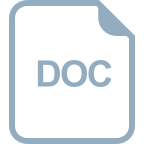








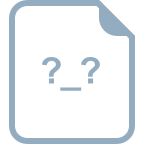