C++设计一个程序要求(1)输入某班级学生的姓名、分数;(2)对(1)的分数进行降序排列并输出;(3)具有输入输出界面
时间: 2024-12-08 10:19:26 浏览: 24
为了在C++中设计这样一个程序,我们将需要使用`iostream`库处理输入输出,以及一个简单的数据结构来存储学生的信息,并实现排序功能。这里是一个基础的示例:
```cpp
#include <iostream>
#include <vector>
#include <algorithm> // 使用sort函数
// 定义学生类,包含姓名和分数
class Student {
public:
std::string name;
int score;
// 构造函数
Student(std::string n, int s) : name(n), score(s) {}
};
// 对学生列表进行降序排序
void sortStudents(std::vector<Student>& students) {
std::sort(students.begin(), students.end(), [](const Student& a, const Student& b) { return a.score > b.score; });
}
int main() {
std::vector<Student> studentList;
std::string name;
int score;
// 输入阶段
while (true) {
std::cout << "请输入学生姓名(输入'q'退出): ";
getline(std::cin, name);
if (name == "q") break;
std::cout << "请输入学生的分数: ";
std::cin >> score;
// 检查输入是否有效
if (score < 0 || score > 100) {
std::cerr << "无效分数,已忽略。\n";
continue;
}
studentList.push_back(Student(name, score));
}
// 排序并输出
std::cout << "\n按照分数降序排列:\n";
for (const auto& student : studentList) {
std::cout << "姓名: " << student.name << ", 分数: " << student.score << '\n';
}
sortStudents(studentList);
std::cout << "排序后的成绩:\n";
for (const auto& student : studentList) {
std::cout << "姓名: " << student.name << ", 分数: " << student.score << '\n';
}
return 0;
}
```
在这个程序中,我们首先创建了一个Student类,然后在main函数里通过循环接收用户的输入,将数据添加到`std::vector<Student>`中。之后,我们对这个学生列表进行降序排序,并分别在输入和排序后输出。
阅读全文
相关推荐

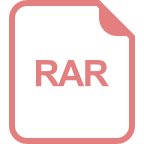
















