详细说明怎么快速测试增加节点和删除节点在红黑树的所有情况
时间: 2023-03-13 11:41:04 浏览: 85
测试增加节点和删除节点在红黑树中,需要以下几步:1.首先,检查红黑树是否为空;2.如果树为空,则创建一个空节点;3.然后,插入新节点;4.修复树,以确保右黑色节点;5.最后,删除要删除的节点,并重新调整红黑树,确保右黑色节点的性质。
相关问题
用java构建红黑树,并包含节点的增加和删除
红黑树是一种自平衡二叉查找树,其性质如下:
1. 每个节点要么是红色,要么是黑色。
2. 根节点是黑色。
3. 每个叶子节点(NIL节点,空节点)是黑色的。
4. 如果一个节点是红色的,则它的两个子节点都是黑色的。
5. 对于每个节点,从该节点到其所有后代叶子节点的简单路径上,均包含相同数目的黑色节点。
下面是Java代码实现红黑树,包括节点的增加和删除:
```java
public class RedBlackTree {
private final int RED = 0;
private final int BLACK = 1;
private class Node {
int val = -1;
int color = BLACK;
Node left = null;
Node right = null;
Node parent = null;
public Node(int val) {
this.val = val;
}
}
private Node root = null;
public void insert(int val) {
Node newNode = new Node(val);
Node parent = null;
Node node = root;
while (node != null) {
parent = node;
if (val < node.val) {
node = node.left;
} else {
node = node.right;
}
}
newNode.parent = parent;
if (parent == null) {
root = newNode;
} else if (val < parent.val) {
parent.left = newNode;
} else {
parent.right = newNode;
}
insertFixUp(newNode);
}
private void insertFixUp(Node node) {
Node parent, grandParent;
while ((parent = node.parent) != null && parent.color == RED) {
grandParent = parent.parent;
if (parent == grandParent.left) {
Node uncle = grandParent.right;
if (uncle != null && uncle.color == RED) {
parent.color = BLACK;
uncle.color = BLACK;
grandParent.color = RED;
node = grandParent;
} else {
if (node == parent.right) {
leftRotate(parent);
Node temp = parent;
parent = node;
node = temp;
}
parent.color = BLACK;
grandParent.color = RED;
rightRotate(grandParent);
}
} else {
Node uncle = grandParent.left;
if (uncle != null && uncle.color == RED) {
parent.color = BLACK;
uncle.color = BLACK;
grandParent.color = RED;
node = grandParent;
} else {
if (node == parent.left) {
rightRotate(parent);
Node temp = parent;
parent = node;
node = temp;
}
parent.color = BLACK;
grandParent.color = RED;
leftRotate(grandParent);
}
}
}
root.color = BLACK;
}
private void leftRotate(Node node) {
Node right = node.right;
node.right = right.left;
if (right.left != null) {
right.left.parent = node;
}
right.parent = node.parent;
if (node.parent == null) {
root = right;
} else if (node == node.parent.left) {
node.parent.left = right;
} else {
node.parent.right = right;
}
right.left = node;
node.parent = right;
}
private void rightRotate(Node node) {
Node left = node.left;
node.left = left.right;
if (left.right != null) {
left.right.parent = node;
}
left.parent = node.parent;
if (node.parent == null) {
root = left;
} else if (node == node.parent.right) {
node.parent.right = left;
} else {
node.parent.left = left;
}
left.right = node;
node.parent = left;
}
public void delete(int val) {
Node node = search(val);
if (node == null) {
return;
}
Node child, parent;
int color;
if (node.left != null && node.right != null) {
Node replace = node.right;
while (replace.left != null) {
replace = replace.left;
}
if (node.parent != null) {
if (node == node.parent.left) {
node.parent.left = replace;
} else {
node.parent.right = replace;
}
} else {
root = replace;
}
child = replace.right;
parent = replace.parent;
color = replace.color;
if (parent == node) {
parent = replace;
} else {
if (child != null) {
child.parent = parent;
}
parent.left = child;
replace.right = node.right;
node.right.parent = replace;
}
replace.parent = node.parent;
replace.color = node.color;
replace.left = node.left;
node.left.parent = replace;
if (color == BLACK) {
deleteFixUp(child, parent);
}
node = null;
return;
}
if (node.left != null) {
child = node.left;
} else {
child = node.right;
}
parent = node.parent;
color = node.color;
if (child != null) {
child.parent = parent;
}
if (parent != null) {
if (node == parent.left) {
parent.left = child;
} else {
parent.right = child;
}
} else {
root = child;
}
if (color == BLACK) {
deleteFixUp(child, parent);
}
node = null;
}
private void deleteFixUp(Node node, Node parent) {
Node other;
while ((node == null || node.color == BLACK) && node != root) {
if (parent.left == node) {
other = parent.right;
if (other.color == RED) {
other.color = BLACK;
parent.color = RED;
leftRotate(parent);
other = parent.right;
}
if ((other.left == null || other.left.color == BLACK) &&
(other.right == null || other.right.color == BLACK)) {
other.color = RED;
node = parent;
parent = node.parent;
} else {
if (other.right == null || other.right.color == BLACK) {
other.left.color = BLACK;
other.color = RED;
rightRotate(other);
other = parent.right;
}
other.color = parent.color;
parent.color = BLACK;
other.right.color = BLACK;
leftRotate(parent);
node = root;
break;
}
} else {
other = parent.left;
if (other.color == RED) {
other.color = BLACK;
parent.color = RED;
rightRotate(parent);
other = parent.left;
}
if ((other.left == null || other.left.color == BLACK) &&
(other.right == null || other.right.color == BLACK)) {
other.color = RED;
node = parent;
parent = node.parent;
} else {
if (other.left == null || other.left.color == BLACK) {
other.right.color = BLACK;
other.color = RED;
leftRotate(other);
other = parent.left;
}
other.color = parent.color;
parent.color = BLACK;
other.left.color = BLACK;
rightRotate(parent);
node = root;
break;
}
}
}
if (node != null) {
node.color = BLACK;
}
}
private Node search(int val) {
Node node = root;
while (node != null) {
if (node.val == val) {
return node;
} else if (val < node.val) {
node = node.left;
} else {
node = node.right;
}
}
return null;
}
}
```
注:以上代码仅供学习参考,实际使用要根据具体情况进行修改。
Java设计一棵红黑树,方法包括插入节点,删除节点和查找节点
红黑树是一种自平衡二叉查找树,它保证了插入、删除和查找操作的最坏情况时间复杂度为 O(log n)。红黑树的节点有两种颜色,红色和黑色,同时满足以下性质:
1. 根节点为黑色;
2. 所有叶子节点都是黑色;
3. 如果一个节点是红色的,则它的两个子节点都是黑色的;
4. 从任意一个节点到其每个叶子节点的所有路径都包含相同数目的黑色节点;
5. 每个节点的左子树和右子树都是红黑树。
下面是 Java 实现红黑树的代码:
```
public class RedBlackTree<T extends Comparable<T>> {
private Node<T> root;
private static final boolean RED = true;
private static final boolean BLACK = false;
private static class Node<T> {
T value;
Node<T> left, right;
boolean color;
Node(T value, boolean color) {
this.value = value;
this.color = color;
}
}
// 插入节点
public void insert(T value) {
root = insert(root, value);
root.color = BLACK;
}
private Node<T> insert(Node<T> node, T value) {
if (node == null) {
return new Node<>(value, RED);
}
int cmp = value.compareTo(node.value);
if (cmp < 0) {
node.left = insert(node.left, value);
} else if (cmp > 0) {
node.right = insert(node.right, value);
} else {
node.value = value;
}
if (isRed(node.right) && !isRed(node.left)) {
node = rotateLeft(node);
}
if (isRed(node.left) && isRed(node.left.left)) {
node = rotateRight(node);
}
if (isRed(node.left) && isRed(node.right)) {
flipColors(node);
}
return node;
}
// 删除节点
public void delete(T value) {
if (root == null) {
return;
}
if (!isRed(root.left) && !isRed(root.right)) {
root.color = RED;
}
root = delete(root, value);
if (root != null && !isRed(root.left) && !isRed(root.right)) {
root.color = BLACK;
}
}
private Node<T> delete(Node<T> node, T value) {
if (value.compareTo(node.value) < 0) {
if (!isRed(node.left) && !isRed(node.left.left)) {
node = moveRedLeft(node);
}
node.left = delete(node.left, value);
} else {
if (isRed(node.left)) {
node = rotateRight(node);
}
if (value.compareTo(node.value) == 0 && node.right == null) {
return null;
}
if (!isRed(node.right) && !isRed(node.right.left)) {
node = moveRedRight(node);
}
if (value.compareTo(node.value) == 0) {
Node<T> min = findMin(node.right);
node.value = min.value;
node.right = deleteMin(node.right);
} else {
node.right = delete(node.right, value);
}
}
return balance(node);
}
// 查找节点
public Node<T> find(T value) {
Node<T> node = root;
while (node != null) {
int cmp = value.compareTo(node.value);
if (cmp < 0) {
node = node.left;
} else if (cmp > 0) {
node = node.right;
} else {
return node;
}
}
return null;
}
private boolean isRed(Node<T> node) {
return node != null && node.color == RED;
}
private Node<T> rotateLeft(Node<T> node) {
Node<T> x = node.right;
node.right = x.left;
x.left = node;
x.color = node.color;
node.color = RED;
return x;
}
private Node<T> rotateRight(Node<T> node) {
Node<T> x = node.left;
node.left = x.right;
x.right = node;
x.color = node.color;
node.color = RED;
return x;
}
private void flipColors(Node<T> node) {
node.color = RED;
node.left.color = BLACK;
node.right.color = BLACK;
}
private Node<T> moveRedLeft(Node<T> node) {
flipColors(node);
if (isRed(node.right.left)) {
node.right = rotateRight(node.right);
node = rotateLeft(node);
flipColors(node);
}
return node;
}
private Node<T> moveRedRight(Node<T> node) {
flipColors(node);
if (isRed(node.left.left)) {
node = rotateRight(node);
flipColors(node);
}
return node;
}
private Node<T> findMin(Node<T> node) {
while (node.left != null) {
node = node.left;
}
return node;
}
private Node<T> deleteMin(Node<T> node) {
if (node.left == null) {
return null;
}
if (!isRed(node.left) && !isRed(node.left.left)) {
node = moveRedLeft(node);
}
node.left = deleteMin(node.left);
return balance(node);
}
private Node<T> balance(Node<T> node) {
if (isRed(node.right)) {
node = rotateLeft(node);
}
if (isRed(node.left) && isRed(node.left.left)) {
node = rotateRight(node);
}
if (isRed(node.left) && isRed(node.right)) {
flipColors(node);
}
return node;
}
}
```
其中,插入节点和删除节点都需要进行旋转和颜色翻转等操作来保证红黑树的性质。查找节点则是一个简单的二叉查找。
相关推荐
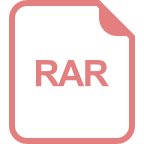
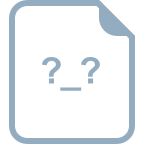
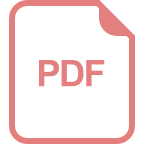
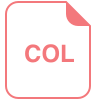
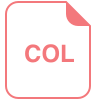
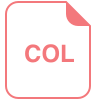
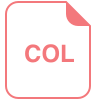
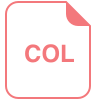







