理想滤波器,高斯滤波器,巴特沃斯滤波器的代码
时间: 2023-07-09 13:09:28 浏览: 106
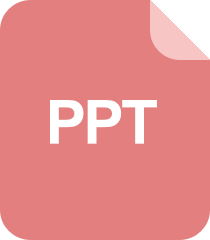
同轴腔体滤波器的设计-微波腔体滤波器的设计
以下是三种常见滤波器的示例代码:
1. 理想滤波器
```python
import numpy as np
import cv2
def ideal_filter(img, d0):
'''
理想低通滤波器
img: 输入图像
d0: 截止频率
'''
# 傅里叶变换
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
# 构造理想低通滤波器
rows, cols = img.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.uint8)
for i in range(rows):
for j in range(cols):
if np.sqrt((i-crow)**2 + (j-ccol)**2) <= d0:
mask[i,j] = 1
# 滤波
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
return img_back.astype(np.uint8)
```
2. 高斯滤波器
```python
import numpy as np
import cv2
def gaussian_filter(img, d0, sigma=1):
'''
高斯低通滤波器
img: 输入图像
d0: 截止频率
sigma: 方差,默认为1
'''
# 傅里叶变换
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
# 构造高斯低通滤波器
rows, cols = img.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.float32)
for i in range(rows):
for j in range(cols):
distance = np.sqrt((i-crow)**2 + (j-ccol)**2)
mask[i,j] = np.exp(-distance**2 / (2*sigma**2)) # 高斯公式
mask = 1 - mask # 反转滤波器
mask = mask.astype(np.float32)
# 滤波
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
return img_back.astype(np.uint8)
```
3. 巴特沃斯滤波器
```python
import numpy as np
import cv2
def butterworth_filter(img, d0, n=1):
'''
巴特沃斯低通滤波器
img: 输入图像
d0: 截止频率
n: 阶数,默认为1
'''
# 傅里叶变换
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
# 构造巴特沃斯低通滤波器
rows, cols = img.shape
crow, ccol = rows//2, cols//2
mask = np.zeros((rows, cols), np.float32)
for i in range(rows):
for j in range(cols):
distance = np.sqrt((i-crow)**2 + (j-ccol)**2)
mask[i,j] = 1 / (1 + (distance/d0)**(2*n)) # 巴特沃斯公式
mask = 1 - mask # 反转滤波器
mask = mask.astype(np.float32)
# 滤波
fshift = fshift * mask
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.abs(img_back)
return img_back.astype(np.uint8)
```
阅读全文
相关推荐
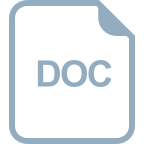
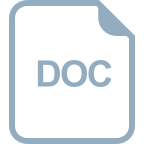

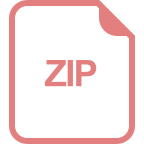
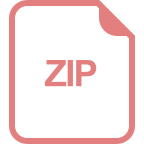
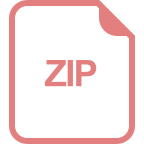
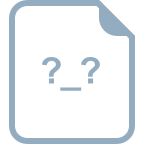










