CShap 二叉树节点的所有祖先节点 源代码
时间: 2023-12-01 19:01:22 浏览: 91
下面是C#实现二叉树节点的所有祖先节点的源代码:
```csharp
using System;
using System.Collections.Generic;
namespace BinaryTreeAncestor
{
public class Node
{
public int Value { get; set; }
public Node LeftChild { get; set; }
public Node RightChild { get; set; }
public Node(int value)
{
Value = value;
}
//获取当前节点的所有祖先节点
public List<Node> GetAncestors()
{
List<Node> ancestors = new List<Node>();
Node current = this;
while (current != null)
{
ancestors.Add(current);
current = current.Parent;
}
return ancestors;
}
//获取当前节点的父节点
public Node Parent { get; set; }
}
class Program
{
static void Main(string[] args)
{
Node root = new Node(1)
{
LeftChild = new Node(2)
{
LeftChild = new Node(4),
RightChild = new Node(5)
},
RightChild = new Node(3)
{
LeftChild = new Node(6),
RightChild = new Node(7)
}
};
List<Node> ancestors = root.LeftChild.RightChild.GetAncestors();
Console.WriteLine("节点{0}的祖先节点为:", root.LeftChild.RightChild.Value);
foreach (Node node in ancestors)
{
Console.Write(node.Value + " ");
}
Console.ReadLine();
}
}
}
```
在该实现中,我们为节点类添加了一个`Parent`属性,表示当前节点的父节点。然后在`GetAncestors`方法中,我们使用一个`while`循环来遍历当前节点的所有祖先节点,每次将当前节点加入到祖先节点列表中,并将当前节点更新为其父节点,直到当前节点为`null`(即已经遍历到根节点)。最后,在`Main`方法中,我们测试了获取二叉树中某个节点的所有祖先节点的功能。
阅读全文
相关推荐






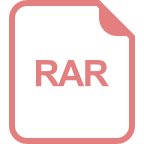







