CShap 二叉树所有根到叶的路径 源代码
时间: 2023-11-14 08:13:10 浏览: 85
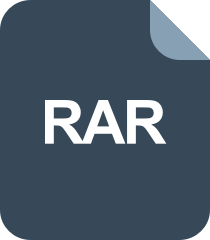
二叉树的源代码
下面是 C# 的二叉树所有根到叶的路径的源代码:
```csharp
using System;
using System.Collections.Generic;
public class TreeNode {
public int val;
public TreeNode left;
public TreeNode right;
public TreeNode(int x) { val = x; }
}
public class Solution {
public IList<string> BinaryTreePaths(TreeNode root) {
List<string> res = new List<string>();
if (root == null) return res;
DFS(root, "", res);
return res;
}
private void DFS(TreeNode node, string path, List<string> res) {
if (node.left == null && node.right == null) {
res.Add(path + node.val);
return;
}
if (node.left != null) DFS(node.left, path + node.val + "->", res);
if (node.right != null) DFS(node.right, path + node.val + "->", res);
}
}
```
这段代码使用深度优先搜索(DFS)算法,对二叉树进行遍历,找出所有根节点到叶节点的路径。具体实现过程如下:
1. 如果当前节点为叶节点,将当前路径和节点值拼接起来,加入结果列表中。
2. 如果当前节点有左子树,递归遍历左子树,并将当前节点值拼接到路径中。
3. 如果当前节点有右子树,递归遍历右子树,并将当前节点值拼接到路径中。
最后将所有路径组成的列表返回即可。
阅读全文
相关推荐














