#include<stdio.h> #include<malloc.h> #define OK 1 #define ERROR 0 #define OVERFLOW -2 typedef int Status; typedef int ElemType ; typedef struct node { ElemType data; struct node *node; } Status InitList(LinkList *l) { *l=(linklist)malloc(sizeof(node)); (*l)-next=null; } void createlist(LinkList l) { node *s; char c; int flag=1; while(flag) { c=getchar(); if(c!='s') } s=(node *)malloc(sizeof(node)); s->data=c; s->next=l->next; l->next=s; } else flag=0; } node* get data(linklist l,int i) { int j; node *p; if(i<=0)return null; p=l ;j=0; while((p-next!=null)&&(j<i)) { p=p->next; j++; } if(i==j) return p; else return null; } int main(void) { int n, LinkList L; printf("输入要创建的单链表的元素个数\n"); scanf("%d",&n); printf("请输入各个元素 \n"); CreateList(&L,n); printf("创建的单链表成功\n"); printf("第二个元素值:%d\n",L->next->next->data); printf("请输入要查找的数据:\n"); scanf("%d",&e); if(LocateElem(L,e)==NULL) printf("查找失败\n"); else printf("数据所在位置为%d\n",LocateElem(L,e)); return 0; }
时间: 2023-12-09 20:03:51 浏览: 32
这段代码存在一些语法错误,我先列举一下:
1. 函数 `InitList` 的返回类型应该是 `Status`,而不是 `void`。
2. 在 `InitList` 函数中,链表头节点指针的 `next` 字段应该是 `NULL`,而不是 `null`。
3. 函数 `createlist` 中的 `node` 应该是 `node *`。
4. 函数 `createlist` 中的 `l` 应该是 `LinkList` 类型,而不是 `LinkList` 的指针类型。
5. 函数 `get data` 中的 `null` 应该是 `NULL`。
6. 函数 `main` 中的 `CreateList` 应该是 `createlist`。
7. 函数 `main` 中的 `e` 没有定义。
除此之外,还有一些问题需要注意:
1. 函数 `InitEND;
注意:以上SQL语句仅供参考,具体实现可能需要根据实际情况进行调整。
相关问题
#include <stdio.h> #include <stdlib.h> #define MAXSIZE 100 typedef int ElemType; typedef struct
这段代码是C语言中的头文件引用和一些定义的示例。其中,`#include <stdio.h>`和`#include <stdlib.h>`是用来引入标准库函数的头文件。`stdio.h`包含了输入输出相关的函数,如`printf`和`scanf`;`stdlib.h`包含了一些常用的函数,如内存分配函数`malloc`和随机数生成函数`rand`。
`#define MAXSIZE 100`是一个宏定义,用来定义一个常量`MAXSIZE`,它的值为100。
`typedef int ElemType;`是用来给`int`类型起一个别名`ElemType`,方便在代码中使用。
至于代码中的结构体部分,由于你没有提供完整的代码,所以无法给出具体的解释。但一般来说,结构体用于定义一种自定义的数据类型,可以包含多个不同类型的成员变量。
补全#include<stdio.h> #include<malloc.h> #define MaxSize 50 typedef int ElemType; typedef struct { Elem
#include<stdio.h>
#include<malloc.h>
#define MaxSize 50
typedef int ElemType;
typedef struct
{
ElemType data[MaxSize];
int length;
}SqList;
这段代码是一个C语言的头文件补全,包含了stdio.h和malloc.h两个头文件,并定义了一个常量MaxSize为50。同时,定义了一个类型别名ElemType为int,并声明了一个结构体SqList,其中包含了一个数组data和一个整型变量length。
相关推荐
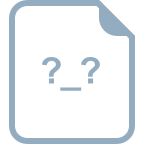
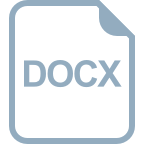
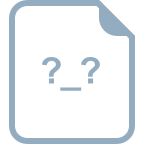














