c语言数据结构完整代码以字符序列的形式从终端输入语法正确、不含变量的表达式,表示算符优先关系,实现算法四则混合运算表达式求值
时间: 2024-11-27 09:21:54 浏览: 21
在C语言中,我们可以创建一个简单的解析器来处理这种四则运算的表达式输入。这里是一个基本的示例,它使用递归下降分析法来解析输入并计算结果。请注意,这只是一个基础版本,未包含错误检查和完整的优化:
```c
#include <stdio.h>
#include <ctype.h>
// 定义操作数和栈结构体
typedef int Operand;
typedef struct {
char operator; // 操作符
Operand left; // 左操作数
Operand right; // 右操作数
} Token;
// 计算操作结果
Operand calculate(Operand a, char op, Operand b) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
if (b != 0)
return a / b;
else
printf("Error: Division by zero.\n");
return 0; // 返回0防止程序崩溃
default:
printf("Invalid operator.\n");
return 0;
}
}
// 解析函数
int evaluate(char* expression) {
char ch;
Stack<Token> stack;
bool is_number = false;
Operand num = 0;
while ((ch = getchar()) != EOF) {
if (isdigit(ch)) {
is_number = true;
num = num * 10 + ch - '0';
} else if (is_number) {
stack.push({'.', num, 0});
is_number = false;
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
if (!stack.empty()) {
stack.top().right = num;
num = calculate(stack.top().left, ch, stack.pop().right);
stack.push({ch, stack.top().left, num});
} else {
stack.push({'.', num, 0});
}
} else if (ch == '(') {
stack.push({ch, 0, 0});
} else if (ch == ')') {
if (stack.empty() || stack.top().operator != '(') {
printf("Error: Unmatched parentheses.\n");
return 0;
}
while (!stack.empty() && stack.top().operator != '(') {
num = calculate(stack.top().left, stack.top().operator, stack.pop().right);
stack.push({stack.top().operator, stack.top().left, num});
}
if (stack.top().operator == '(') {
stack.pop();
}
}
}
if (!stack.empty()) {
printf("Error: Unmatched parentheses.\n");
return 0;
} else {
return stack.top().right;
}
}
int main() {
char input[100];
printf("Enter an arithmetic expression without variables: ");
fgets(input, sizeof(input), stdin);
// 去掉换行符
input[strlen(input) - 1] = '\0';
if (evaluate(input)) {
printf("Result: %d\n", evaluate(input));
}
return 0;
}
```
阅读全文
相关推荐
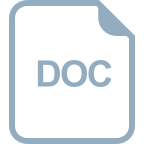
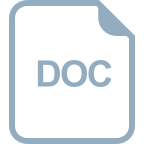
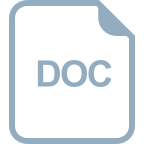



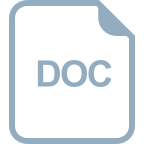
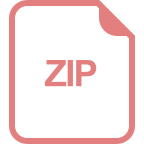
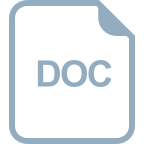
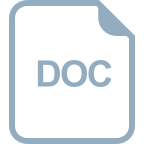
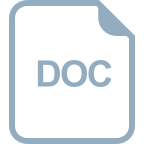
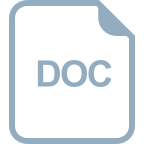
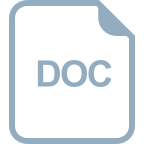
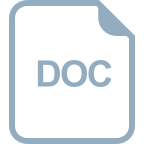
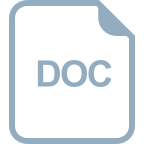
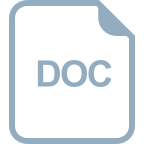
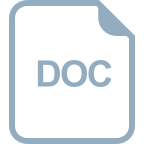
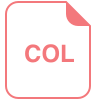
