用c++对已建立的单向链表进行插入、删除、查找等基本操作,并用多组数据进行测试写完整代码
时间: 2024-10-20 09:09:33 浏览: 35
在C++中,我们可以创建一个简单的单向链表结构来实现插入、删除和查找操作。首先,我们需要定义节点(Node)结构和链表(LinkedList)类。以下是基础操作的代码示例:
```cpp
#include <iostream>
// 定义链表节点
struct Node {
int data;
Node* next; // 指向下一个节点的指针
Node(int value) : data(value), next(nullptr) {}
};
// 链表类
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
// 插入节点到链表头部
void insertAtFront(int value) {
Node* newNode = new Node(value);
newNode->next = head;
head = newNode;
}
// 插入节点到链表尾部
void insertAtEnd(int value) {
if (!head) {
head = new Node(value);
return;
}
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = new Node(value);
}
// 查找特定值并返回其所在的节点位置(如果存在)
Node* find(int value) {
Node* current = head;
for (; current; current = current->next) {
if (current->data == value) {
return current;
}
}
return nullptr; // 如果找不到,返回nullptr
}
// 删除指定值的第一个匹配项
void remove(int value) {
if (!head || head->data != value) return;
Node* temp = head;
head = head->next;
delete temp;
}
// 打印链表中的所有元素
void display() {
Node* current = head;
while (current) {
std::cout << current->data << " ";
current = current->next;
}
std::cout << std::endl;
}
};
int main() {
LinkedList list;
// 测试插入操作
list.insertAtFront(5);
list.insertAtFront(3);
list.insertAtEnd(8);
// 打印链表
list.display(); // 输出:3 5 8
// 测试查找操作
Node* foundNode = list.find(5); // 找到值为5的节点
if (foundNode) {
std::cout << "Found node with value: " << foundNode->data << std::endl;
} else {
std::cout << "Value not found." << std::endl;
}
// 测试删除操作
list.remove(5); // 删除值为5的节点
// 再次打印链表
list.display(); // 输出:3 8
return 0;
}
```
这段代码展示了如何使用C++实现单向链表的基本操作。你可以根据需要修改或扩展它,例如添加更多复杂的数据结构或错误处理功能。
阅读全文
相关推荐
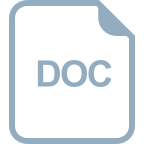
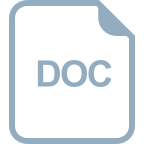
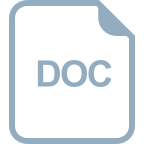



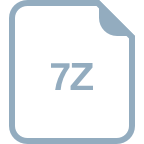
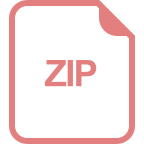
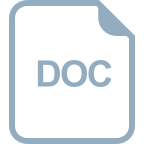
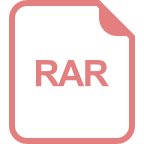
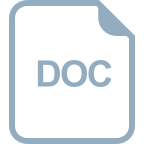
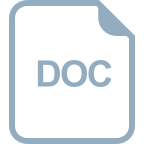
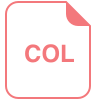
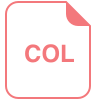
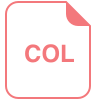
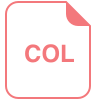
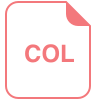
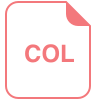
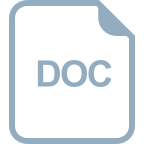