对已建立的单链表实现插入、删除等基本操作c++。
时间: 2024-09-24 12:13:52 浏览: 43
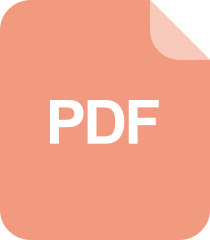
C++中单链表的建立与基本操作
在C++中,对已建立的单链表实现插入和删除操作主要包括以下几种情况:
### 插入操作:
#### 1. **在链表尾部插入**
- 需要一个指针`tail`来跟踪链表的最后一个节点。如果链表为空,直接创建一个新的节点作为头结点;否则,先创建新节点然后将其`next`指向当前尾节点,最后更新`tail`为新节点。
```cpp
void insertAtEnd(Node*& head, Node*& tail, int value) {
if (head == nullptr) {
head = new Node(value);
tail = head;
} else {
Node* newNode = new Node(value);
tail->next = newNode;
tail = newNode; // 如果新节点是最后一个,也需要更新tail
}
}
```
#### 2. **在指定位置插入**
- 可以使用两个指针分别指向目标插入位置的前一个节点和当前节点,然后将新节点连接在这两者之间。
```cpp
void insertAfter(Node*& current, Node*& head, int value) {
Node* newNode = new Node(value);
newNode->next = current->next;
current->next = newNode;
}
```
### 删除操作:
#### 1. **删除某个值的所有节点**
- 使用双向链表可以更方便地查找和删除节点,因为每个节点都有一个`prev`指向前一个节点的引用。遍历链表,找到第一个匹配的节点并删除它。
```cpp
void removeByValue(Node*& head, int value) {
if (head == nullptr) return;
Node* current = head;
while (current != nullptr && current->data != value) {
current = current->next;
}
if (current != nullptr) { // 找到了值为value的节点
if (current->prev != nullptr) {
current->prev->next = current->next;
} else { // 如果是头节点
head = current->next;
}
if (current->next != nullptr) {
current->next->prev = current->prev;
}
delete current;
}
}
```
#### 2. **删除某个特定位置的节点**
- 类似于插入操作,找到目标位置的前一个节点,然后修改它的`next`指针跳过要删除的节点。
```cpp
void removeAtPosition(Node*& head, int position) {
if (position < 0 || head == nullptr) return;
Node* current = head;
for (int i = 0; i < position; ++i) {
if (current == nullptr) break;
current = current->next;
}
if (current != nullptr && current->next != nullptr) {
current->next = current->next->next;
}
}
```
阅读全文
相关推荐
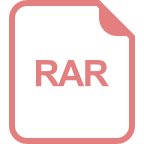
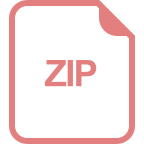
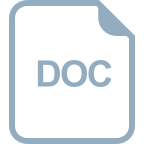








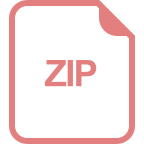
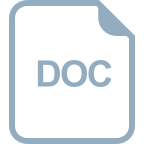
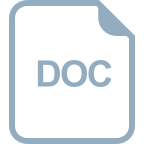



