解释代码def toCreateTable(self): cursor = self.conn.cursor() cursor.execute("create table IF NOT EXISTS person(id INTEGER primary key autoincrement, personName varchar(60),personPwd varchar(60))")
时间: 2024-04-20 14:24:02 浏览: 15
这段代码是一个Python函数,用于创建一个名为"person"的表格(table)。
首先,通过`self.conn.cursor()`创建了一个游标(cursor)对象,用于执行SQL语句。
然后,调用`cursor.execute()`方法执行SQL语句。在这里,执行的是一个CREATE TABLE语句,用于创建一个名为"person"的表格。
CREATE TABLE语句的具体内容是:"create table IF NOT EXISTS person(id INTEGER primary key autoincrement, personName varchar(60),personPwd varchar(60))"。
这个语句指定了表格的结构和列的属性。该表格包含了三列:id、personName和personPwd。其中,id列是主键(PRIMARY KEY),并且使用了autoincrement关键字表示自动递增。personName和personPwd列都是varchar类型,并且限制了最大长度为60个字符。
在CREATE TABLE语句中,还使用了IF NOT EXISTS关键字,表示如果表格不存在才创建。这样可以避免重复创建表格导致的错误。
通过执行这段代码,可以创建一个名为"person"的表格,并定义了相应的列和属性。
相关问题
解释代码 class dbUtil(): def __init__(self): conn, cursor = self.get_conn() self.conn = conn self.cursor = cursor def get_time(self): time_str = time.strftime("%Y{}%m{}%d{} %X") return time_str.format("年", "月", "日") def get_conn(self): # 建立连接 conn = pymysql.connect(host="127.0.0.1", user="root", password="123456", db="shopping_analysis", charset="utf8") # c创建游标A cursor = conn.cursor() return conn, cursor def close_commit(self): self.conn.commit() if self.cursor: self.cursor.close() if self.conn: self.conn.close() def close(self): self.conn.commit() if self.cursor: self.cursor.close() if self.conn: self.conn.close() def query(self, sql, *args): self.cursor.execute(sql, args) res = self.cursor.fetchall() return res def query_noargs(self, sql): self.cursor.execute(sql) res = self.cursor.fetchall() return res
这是一个 Python 类 `dbUtil`,用于连接数据库和执行 SQL 查询。类中有以下方法:
- `__init__(self)`:类的初始化方法,用于获取连接和游标对象,并将其保存到 `self.conn` 和 `self.cursor` 属性中。
- `get_time(self)`:获取当前时间的方法,返回格式化后的时间字符串。
- `get_conn(self)`:获取数据库连接和游标对象的方法,返回 `conn` 和 `cursor` 对象。
- `close_commit(self)`:关闭连接并提交事务的方法。
- `close(self)`:关闭连接的方法。
- `query(self, sql, *args)`:执行带有参数的 SQL 查询并返回结果集。
- `query_noargs(self, sql)`:执行不带参数的 SQL 查询并返回结果集。
import tkinter as tk import sqlite3 import random from tkinter import messagebox root = tk.Tk() app = EnglishLearningApp(root) root.mainloop() class EnglishLearningApp: def __init__(self, master): self.master = master master.title("英语学习软件") self.database_button = tk.Button(master, text="单词库", command=self.word_consult) self.database_button.pack(pady=10) self.quiz_button = tk.Button(master, text="英语默写", command=self.quiz_page) self.quiz_button.pack(pady=10) self.conn = sqlite3.connect('words.db') self.cursor = self.conn.cursor() self.cursor.execute('''CREATE TABLE IF NOT EXISTS words (word text PRIMARY KEY)''') self.conn.commit() def word_consult(self): self.word_entry = tk.Entry(self.word_consult) self.word_entry.pack(padx=10, pady=10) self.add_button = tk.Button(self.word_consult, text="添加/删除单词", command=self.add_word) self.add_button.pack(pady=5) name = search_entry.get() cur.execute("SELECT * FROM data WHERE name=?", (name,)) word = cur.fetchone() if word: self.cursor.execute("INSERT INTO words VALUES (?)", (word,)) self.conn.commit() else: self.cursor.execute("DELETE FROM words WHERE word=?", (word,)) self.conn.commit()
这段代码存在一些问题:
1. EnglishLearningApp 类没有定义完整,缺少 quiz_page 函数实现。
2. 在 word_consult 函数中,创建了一个名为 word_entry 的 Entry 对象,但是它没有被放置到任何容器中,应该使用 self.word_entry。
3. 在 add_word 函数中,使用了 search_entry.get(),但是没有定义 search_entry 对象,应该使用 self.word_entry.get()。
4. 在 add_word 函数中,使用了 cur.execute(),但是应该使用 self.cursor.execute()。
5. 在 add_word 函数中,应该把获取的 word 对象转换为字符串,即使用 word[0]。
6. 在 add_word 函数中,应该加入 try-except 结构,以处理插入或删除单词时可能发生的异常情况。
下面是修改后的代码:
```
import tkinter as tk
import sqlite3
import random
from tkinter import messagebox
class EnglishLearningApp:
def __init__(self, master):
self.master = master
master.title("英语学习软件")
self.database_button = tk.Button(master, text="单词库", command=self.word_consult)
self.database_button.pack(pady=10)
self.quiz_button = tk.Button(master, text="英语默写", command=self.quiz_page)
self.quiz_button.pack(pady=10)
self.conn = sqlite3.connect('words.db')
self.cursor = self.conn.cursor()
self.cursor.execute('''CREATE TABLE IF NOT EXISTS words
(word text PRIMARY KEY)''')
self.conn.commit()
def word_consult(self):
self.word_entry = tk.Entry(self.master)
self.word_entry.pack(padx=10, pady=10)
self.add_button = tk.Button(self.master, text="添加/删除单词", command=self.add_word)
self.add_button.pack(pady=5)
def add_word(self):
word = self.word_entry.get()
if not word:
return
try:
self.cursor.execute("SELECT * FROM data WHERE name=?", (word,))
word = self.cursor.fetchone()
if word:
self.cursor.execute("INSERT INTO words VALUES (?)", (str(word[0]),))
self.conn.commit()
messagebox.showinfo("提示", "添加成功")
else:
self.cursor.execute("DELETE FROM words WHERE word=?", (word[0],))
self.conn.commit()
messagebox.showinfo("提示", "删除成功")
except Exception as e:
messagebox.showerror("错误", str(e))
def quiz_page(self):
pass
if __name__ == '__main__':
root = tk.Tk()
app = EnglishLearningApp(root)
root.mainloop()
```
相关推荐
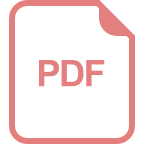
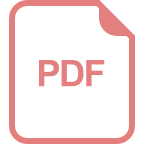












