C#进行图像二值化
时间: 2023-07-04 16:15:43 浏览: 75
在C#中进行图像二值化,可以使用以下步骤:
1. 读取图像:使用Bitmap类加载要处理的图像。
2. 转换为灰度图像:将加载的图像转换为灰度图像,方便后续处理。可以使用ColorMatrix类进行转换。
3. 进行二值化:将灰度图像中的像素灰度值与一个阈值进行比较,将灰度值大于阈值的像素设置为白色,小于阈值的像素设置为黑色。可以使用Bitmap类的SetPixel方法进行像素操作。
下面是一段示例代码:
```csharp
Bitmap bmp = new Bitmap("image.jpg"); // 读取图像
Bitmap grayBmp = new Bitmap(bmp.Width, bmp.Height); // 创建灰度图像
float[][] colorMatrixElements = { new float[] { 0.299f, 0.299f, 0.299f, 0, 0 },
new float[] { 0.587f, 0.587f, 0.587f, 0, 0 },
new float[] { 0.114f, 0.114f, 0.114f, 0, 0 },
new float[] { 0, 0, 0, 1, 0 },
new float[] { 0, 0, 0, 0, 1 } };
ColorMatrix colorMatrix = new ColorMatrix(colorMatrixElements); // 创建灰度转换矩阵
using (Graphics g = Graphics.FromImage(grayBmp))
{
g.DrawImage(bmp, new Rectangle(0, 0, grayBmp.Width, grayBmp.Height),
0, 0, bmp.Width, bmp.Height, GraphicsUnit.Pixel, new ImageAttributes()
{ ColorMatrix = colorMatrix }); // 转换为灰度图像
}
int threshold = 128; // 设置阈值
for (int x = 0; x < grayBmp.Width; x++)
{
for (int y = 0; y < grayBmp.Height; y++)
{
Color color = grayBmp.GetPixel(x, y);
int gray = (int)(color.R * 0.299 + color.G * 0.587 + color.B * 0.114); // 计算灰度值
Color newColor = gray > threshold ? Color.White : Color.Black; // 判断二值化结果
grayBmp.SetPixel(x, y, newColor); // 设置像素颜色
}
}
grayBmp.Save("binary.jpg"); // 保存二值化结果
```
以上代码中,将灰度值大于128的像素设置为白色,小于等于128的像素设置为黑色。可以根据实际情况调整阈值。
相关推荐
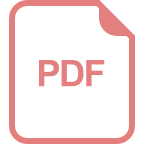
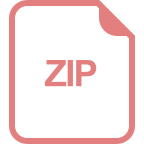
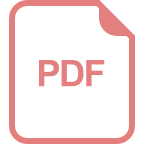














