PIC18F软件模拟IIC读取PCA8565
时间: 2023-11-12 07:06:18 浏览: 163
首先,需要了解一下PCA8565的I2C通信协议。PCA8565是一个实时时钟,它通过I2C接口与微控制器进行通信。在I2C通信中,有两个线路:SCL(时钟线)和SDA(数据线)。SCL由主设备控制,而SDA可以由主设备或从设备控制。每个I2C设备都有一个唯一的7位地址,它用于与主设备通信。
现在,我们来看一下如何在PIC18F微控制器上实现软件模拟I2C通信,以读取PCA8565实时时钟的时间。
首先,需要定义一些常量和变量:
```c
#define SDA PORTCbits.RC4 // SDA 端口
#define SCL PORTCbits.RC3 // SCL 端口
unsigned char PCA8565_ADDRESS = 0xA2; // PCA8565 的 I2C 地址
unsigned char PCA8565_TIME_REGISTER = 0x02; // PCA8565 的时间寄存器地址
unsigned char read_byte = 0; // 读取的字节
unsigned char bit_counter = 0; // 位计数器
unsigned char ack_bit = 0; // 应答位
unsigned char i = 0; // 循环计数器
```
接着,需要编写一些函数来模拟I2C通信中的各个步骤:
1. 发送起始位
```c
void i2c_start(void) {
SDA = 1;
SCL = 1;
__delay_us(5);
SDA = 0;
__delay_us(5);
SCL = 0;
}
```
2. 发送停止位
```c
void i2c_stop(void) {
SDA = 0;
SCL = 1;
__delay_us(5);
SDA = 1;
__delay_us(5);
}
```
3. 发送一个位
```c
void i2c_send_bit(unsigned char bit_value) {
SDA = bit_value;
SCL = 1;
__delay_us(5);
SCL = 0;
}
```
4. 读取一个位
```c
unsigned char i2c_read_bit(void) {
unsigned char bit_value;
SDA = 1;
SCL = 1;
__delay_us(5);
bit_value = SDA;
SCL = 0;
return bit_value;
}
```
5. 发送一个字节
```c
void i2c_send_byte(unsigned char send_byte) {
for (bit_counter = 0; bit_counter < 8; bit_counter++) {
i2c_send_bit((send_byte & 0x80) >> 7);
send_byte <<= 1;
}
ack_bit = i2c_read_bit();
}
```
6. 读取一个字节
```c
unsigned char i2c_read_byte(void) {
read_byte = 0;
for (bit_counter = 0; bit_counter < 8; bit_counter++) {
read_byte <<= 1;
read_byte |= i2c_read_bit();
}
i2c_send_bit(ack_bit);
return read_byte;
}
```
最后,我们可以编写主函数来读取PCA8565的时间:
```c
void main() {
TRISCbits.TRISC3 = 1; // SCL 端口设置为输入
TRISCbits.TRISC4 = 1; // SDA 端口设置为输入
i2c_start(); // 发送起始位
i2c_send_byte(PCA8565_ADDRESS); // 发送设备地址
i2c_send_byte(PCA8565_TIME_REGISTER); // 发送时间寄存器地址
i2c_start(); // 再次发送起始位
i2c_send_byte(PCA8565_ADDRESS + 1); // 发送设备地址(读操作)
read_byte = i2c_read_byte(); // 读取时间字节
i2c_stop(); // 发送停止位
// 输出读取的时间
printf("Time: %02x\n", read_byte);
}
```
这样,我们就完成了PIC18F软件模拟I2C读取PCA8565实时时钟的时间的过程。当然,具体的实现还需要根据实际情况进行调整和优化。
阅读全文
相关推荐
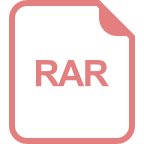
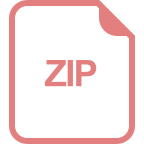

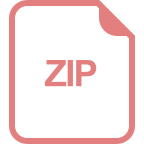
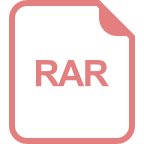
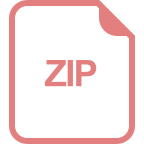
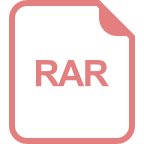
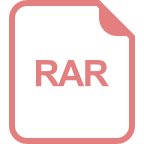
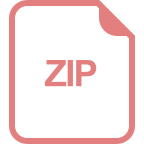
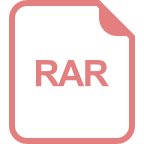
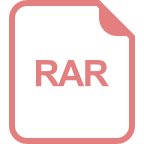



