编程计算球、圆柱和圆锥的表面积和体积。 要求: (1)定义一个基类,至少含有一个数据成员半径,并设为保护成员; (2)定义基类的派生类:球类、圆柱类、圆锥类,都含有求表面积和体积的成员函数和输出函数; (3)编写主函数,求球、圆柱、圆锥的表面积和体积。
时间: 2024-01-22 10:20:08 浏览: 40
以下是一个实现上述要求的 C++ 代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Shape {
protected:
double radius;
public:
Shape(double r): radius(r) {}
virtual double getSurfaceArea() = 0;
virtual double getVolume() = 0;
virtual void print() = 0;
};
class Sphere : public Shape {
public:
Sphere(double r): Shape(r) {}
double getSurfaceArea() {
return 4 * M_PI * pow(radius, 2);
}
double getVolume() {
return 4.0 / 3.0 * M_PI * pow(radius, 3);
}
void print() {
cout << "Sphere with radius " << radius << ":" << endl;
cout << "Surface area = " << getSurfaceArea() << endl;
cout << "Volume = " << getVolume() << endl;
}
};
class Cylinder : public Shape {
protected:
double height;
public:
Cylinder(double r, double h): Shape(r), height(h) {}
double getSurfaceArea() {
return 2 * M_PI * radius * (radius + height);
}
double getVolume() {
return M_PI * pow(radius, 2) * height;
}
void print() {
cout << "Cylinder with radius " << radius << " and height " << height << ":" << endl;
cout << "Surface area = " << getSurfaceArea() << endl;
cout << "Volume = " << getVolume() << endl;
}
};
class Cone : public Cylinder {
public:
Cone(double r, double h): Cylinder(r, h) {}
double getSurfaceArea() {
return M_PI * radius * (radius + sqrt(pow(height, 2) + pow(radius, 2)));
}
double getVolume() {
return 1.0 / 3.0 * M_PI * pow(radius, 2) * height;
}
void print() {
cout << "Cone with radius " << radius << " and height " << height << ":" << endl;
cout << "Surface area = " << getSurfaceArea() << endl;
cout << "Volume = " << getVolume() << endl;
}
};
int main() {
Sphere s(5);
s.print();
Cylinder c(3, 4);
c.print();
Cone k(2, 6);
k.print();
return 0;
}
```
在该代码中,我们首先定义了一个基类 `Shape`,其中包括一个保护成员变量 `radius`,并且定义了三个纯虚函数:`getSurfaceArea()`、`getVolume()` 和 `print()`。这里利用了多态性,让派生类实现这三个函数。
接着,我们定义了三个派生类:`Sphere`、`Cylinder` 和 `Cone`,它们都继承自 `Shape`,并且分别实现了基类中的三个纯虚函数。
最后,在 `main()` 函数中创建了一个 `Sphere` 对象、一个 `Cylinder` 对象和一个 `Cone` 对象,并分别调用它们的 `print()` 函数输出表面积和体积。
相关推荐
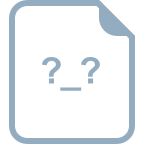
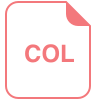








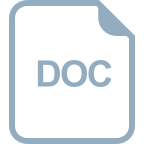
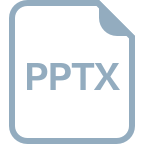
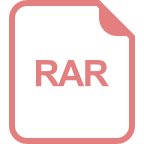
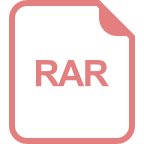
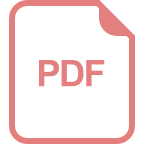
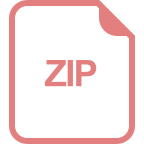