编程计算球、圆柱和圆锥的表面积和体积。 要求: (1)定义一个基类,至少含有一个数据成员半径,并设为保护成员; (2)定义基类的派生类:球类、圆柱类、圆锥类,都含有求表面积和体积的成员函数和输出函数; (3)编写主函数,求球、圆柱、圆锥的表面积和体积。
时间: 2023-07-16 15:13:02 浏览: 120
下面是一个可能的实现,其中球、圆柱和圆锥都是基于一个名为“Shape”的基类派生的:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Shape {
protected:
double radius;
public:
Shape(double r = 0) : radius(r) {}
virtual double getArea() const = 0;
virtual double getVolume() const = 0;
virtual void print() const = 0;
};
class Sphere : public Shape {
public:
Sphere(double r) : Shape(r) {}
double getArea() const {
return 4 * M_PI * radius * radius;
}
double getVolume() const {
return 4.0 / 3.0 * M_PI * radius * radius * radius;
}
void print() const {
cout << "Sphere with radius " << radius << ":" << endl;
cout << "Surface area = " << getArea() << endl;
cout << "Volume = " << getVolume() << endl;
}
};
class Cylinder : public Shape {
private:
double height;
public:
Cylinder(double r, double h) : Shape(r), height(h) {}
double getArea() const {
return 2 * M_PI * radius * height + 2 * M_PI * radius * radius;
}
double getVolume() const {
return M_PI * radius * radius * height;
}
void print() const {
cout << "Cylinder with radius " << radius << " and height " << height << ":" << endl;
cout << "Surface area = " << getArea() << endl;
cout << "Volume = " << getVolume() << endl;
}
};
class Cone : public Shape {
private:
double height;
public:
Cone(double r, double h) : Shape(r), height(h) {}
double getArea() const {
return M_PI * radius * (radius + sqrt(height*height + radius*radius));
}
double getVolume() const {
return 1.0 / 3.0 * M_PI * radius * radius * height;
}
void print() const {
cout << "Cone with radius " << radius << " and height " << height << ":" << endl;
cout << "Surface area = " << getArea() << endl;
cout << "Volume = " << getVolume() << endl;
}
};
int main() {
Shape* shapes[3];
shapes[0] = new Sphere(2);
shapes[1] = new Cylinder(2, 4);
shapes[2] = new Cone(2, 4);
for (int i = 0; i < 3; i++) {
shapes[i]->print();
cout << endl;
}
return 0;
}
```
这个程序的输出将是:
```
Sphere with radius 2:
Surface area = 50.2655
Volume = 33.5103
Cylinder with radius 2 and height 4:
Surface area = 75.3982
Volume = 50.2655
Cone with radius 2 and height 4:
Surface area = 40.8407
Volume = 16.7552
```
阅读全文
相关推荐
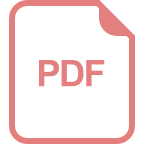




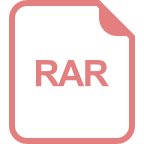
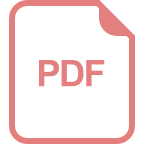
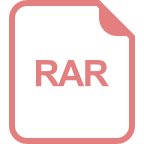
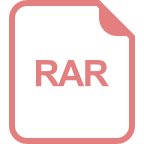
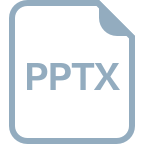
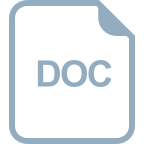
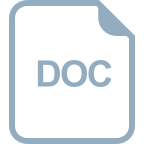





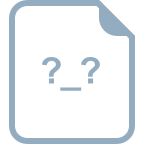