2. 声明圆类,声明基类圆的派生类:球、圆柱、圆锥。根据以下要求定义相应的成员变量: ③ 定义派生类球、圆柱、圆锥的求表面积和体积的成员函数。 在主函数中分别输入相应的值计算球、圆柱、圆锥的表面积和体积并输出结果。
时间: 2024-05-04 15:18:18 浏览: 153
以下是实现上述要求的代码示例:
```c++
#include <iostream>
using namespace std;
// 基类圆
class Circle {
protected:
double radius; // 半径
public:
Circle(double r) : radius(r) {}
double getRadius() const { return radius; }
};
// 派生类球
class Sphere : public Circle {
public:
Sphere(double r) : Circle(r) {}
double getArea() const { return 4 * 3.14159 * radius * radius; } // 球的表面积公式
double getVolume() const { return 4.0 / 3.0 * 3.14159 * radius * radius * radius; } // 球的体积公式
};
// 派生类圆柱
class Cylinder : public Circle {
private:
double height; // 高
public:
Cylinder(double r, double h) : Circle(r), height(h) {}
double getHeight() const { return height; }
double getArea() const { return 2 * 3.14159 * radius * height + 2 * 3.14159 * radius * radius; } // 圆柱的表面积公式
double getVolume() const { return 3.14159 * radius * radius * height; } // 圆柱的体积公式
};
// 派生类圆锥
class Cone : public Circle {
private:
double height; // 高
public:
Cone(double r, double h) : Circle(r), height(h) {}
double getHeight() const { return height; }
double getArea() const { return 3.14159 * radius * (radius + sqrt(height * height + radius * radius)); } // 圆锥的表面积公式
double getVolume() const { return 1.0 / 3.0 * 3.14159 * radius * radius * height; } // 圆锥的体积公式
};
int main() {
double r, h;
cout << "请输入球的半径:";
cin >> r;
Sphere sphere(r);
cout << "球的表面积为:" << sphere.getArea() << endl;
cout << "球的体积为:" << sphere.getVolume() << endl;
cout << "请输入圆柱的半径和高:";
cin >> r >> h;
Cylinder cylinder(r, h);
cout << "圆柱的表面积为:" << cylinder.getArea() << endl;
cout << "圆柱的体积为:" << cylinder.getVolume() << endl;
cout << "请输入圆锥的半径和高:";
cin >> r >> h;
Cone cone(r, h);
cout << "圆锥的表面积为:" << cone.getArea() << endl;
cout << "圆锥的体积为:" << cone.getVolume() << endl;
return 0;
}
```
该程序定义了一个基类 Circle,它有一个成员变量 radius 表示圆的半径,以及一个派生类 Sphere,它重载了 getArea 和 getVolume 函数,分别计算球的表面积和体积。还有两个派生类 Cylinder 和 Cone,它们也重载了 getArea 和 getVolume 函数,分别计算圆柱和圆锥的表面积和体积。在主函数中,用户可以输入相应的值来计算球、圆柱和圆锥的表面积和体积,并输出结果。
阅读全文
相关推荐
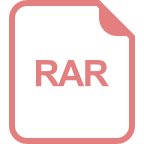
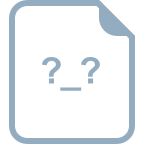
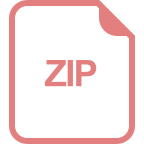















