定义形状类,并派生圆类和矩形类;
时间: 2024-10-10 22:09:28 浏览: 53
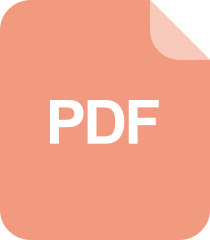
c++ 形状类Shape(派生出圆类Circle和矩形类Rectangle)
在面向对象编程中,我们可以先定义一个抽象的形状类Shape,这个类包含一些通用的几何特性如面积和周长计算方法。然后,我们可以为这种抽象形状创建具体的派生类,例如Circle(圆形)和Rectangle(矩形),它们各自扩展了父类的功能并添加了特有的属性和方法。
```cpp
// 基类 Shape
class Shape {
public:
// 公共属性,如颜色
string color;
// 抽象方法,需要在派生类中具体实现
virtual double getArea() const = 0;
virtual double getCircumference() const = 0;
// 构造函数
Shape(string color) : color(color) {}
protected:
// 析构函数默认不显示
~Shape() {}
private:
};
// 圆形类 Circle,派生自Shape
class Circle : public Shape {
private:
double radius;
public:
Circle(string color, double radius) : Shape(color), radius(radius) {}
// 实现getArea 和 getCircumference方法
double getArea() const override {
return M_PI * radius * radius;
}
double getCircumference() const override {
return 2 * M_PI * radius;
}
};
// 矩形类 Rectangle,派生自Shape
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(string color, double width, double height) : Shape(color), width(width), height(height) {}
// 实现getArea 和 getCircumference方法
double getArea() const override {
return width * height;
}
double getCircumference() const override {
return 2 * (width + height);
}
};
```
在这个例子中,圆形和矩形都重写了基类Shape的虚方法,实现了各自的面积和周长计算,体现了多态性。
阅读全文
相关推荐
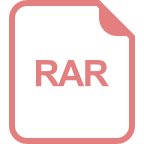
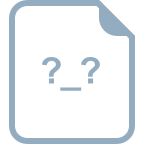















