帮我写一个批量nc转tiff的代码
时间: 2023-08-31 10:40:57 浏览: 165
### 回答1:
为了将多个nc文件批量转换为tiff文件,您可以使用以下Python代码:
```
import os
import gdal
def nc_to_tiff(nc_file, tiff_file):
dataset = gdal.Open(nc_file)
driver = gdal.GetDriverByName("GTiff")
tiff = driver.Create(tiff_file, dataset.RasterXSize, dataset.RasterYSize, dataset.RasterCount, dataset.GetRasterBand(1).DataType)
for i in range(dataset.RasterCount):
band = dataset.GetRasterBand(i+1)
tiff.GetRasterBand(i+1).WriteArray(band.ReadAsArray())
tiff = None
nc_files_dir = "path/to/nc/files"
tiff_files_dir = "path/to/tiff/files"
for nc_file in os.listdir(nc_files_dir):
if nc_file.endswith(".nc"):
nc_to_tiff(os.path.join(nc_files_dir, nc_file), os.path.join(tiff_files_dir, nc_file.replace(".nc", ".tiff")))
```
该代码需要安装GDAL库,您可以通过以下命令安装:
```
pip install gdal
```
您需要替换`nc_files_dir`和`tiff_files_dir`为nc文件和tiff文件的实际路径。
### 回答2:
批量nc转tiff的代码可以使用Python的netCDF4和rasterio库来实现。以下是一个简单的示例代码:
```python
import os
import netCDF4 as nc
import rasterio as rio
# 输入目录和输出目录
input_dir = './input_folder/'
output_dir = './output_folder/'
# 获取输入目录中的所有nc文件
nc_files = [f for f in os.listdir(input_dir) if f.endswith('.nc')]
# 遍历每个nc文件进行转换
for nc_file in nc_files:
# 构建输入和输出文件的路径
input_file = os.path.join(input_dir, nc_file)
output_file = os.path.join(output_dir, os.path.splitext(nc_file)[0] + '.tiff')
# 打开nc文件
dataset = nc.Dataset(input_file)
# 获取变量的数据
variable_data = dataset.variables['variable_name'][:]
# 获取变量的元数据
variable_metadata = dataset.variables['variable_name'].metadata
# 获取变量的维度信息
variable_dimensions = dataset.variables['variable_name'].dimensions
# 关闭nc文件
dataset.close()
# 创建输出tiff文件
with rio.open(output_file, 'w', driver='GTiff', width=variable_data.shape[1], height=variable_data.shape[0], count=1, dtype=variable_data.dtype, crs='EPSG:4326', transform=rio.transform.from_bounds(lon_left, lat_bottom, lon_right, lat_top)) as dst:
# 将nc数据写入tiff文件
dst.write(variable_data, 1)
print('批量转换完成!')
```
请注意,在上述代码中,你需要将`input_folder`替换为保存nc文件的实际输入目录的路径,将`output_folder`替换为保存tiff文件的实际输出目录的路径,将`variable_name`替换为nc文件中你想要转换的变量的名称,还有根据你的数据的具体坐标信息,修改`rio.transform.from_bounds()`中的经纬度范围值。
此代码是一个简单的示例,根据不同的数据集和需求可能需要进行适当的修改和调整。
### 回答3:
要批量将nc文件转换为tiff格式,你可以使用Python语言编写代码。首先,确保你已经安装了Python和GDAL库。以下是一个简单的代码段,可以将当前文件夹中的所有nc文件转换为tiff文件:
```python
import os
from osgeo import gdal
# 获取当前文件夹中所有nc文件的列表
file_list = [f for f in os.listdir('.') if f.endswith('.nc')]
# 遍历文件列表进行转换
for file in file_list:
nc_file = gdal.Open(file) # 打开nc文件
# 从nc文件中读取信息
band = nc_file.GetRasterBand(1)
data = band.ReadAsArray()
# 获取nc文件的空间参考信息
proj = nc_file.GetProjection()
geotransform = nc_file.GetGeoTransform()
# 创建新的tiff文件
tiff_file = file.replace('.nc', '.tiff')
driver = gdal.GetDriverByName('GTiff')
tiff = driver.Create(tiff_file, band.XSize, band.YSize, 1, gdal.GDT_Float32)
# 设置tiff文件的空间参考信息
tiff.SetProjection(proj)
tiff.SetGeoTransform(geotransform)
# 将nc文件中的数据写入tiff文件
output = tiff.GetRasterBand(1)
output.WriteArray(data)
# 关闭文件
nc_file = None
tiff = None
print('批量转换完成!')
```
运行这段代码后,它会自动将当前文件夹中的所有nc文件转换为tiff格式,并保存在同一文件夹下。
请注意,这只是一个简单的示例代码,如果你的nc文件具有多个波段或其他特殊要求,你可能需要进行适当的修改。此外,为了运行这段代码,你需要先安装GDAL库,可以通过`pip install gdal`命令来安装。
阅读全文
相关推荐
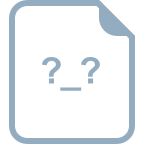
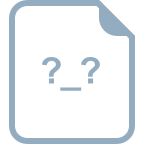


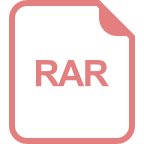
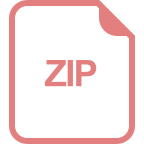
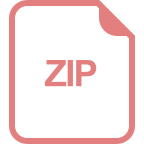




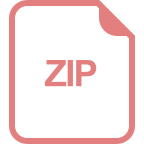