用C语言编写程序根据输入的字符串形式表示的广 义表,创建广义表,然后再输出广义表。 示: 输入:(a,((b,c),d),(#)) 输出:(a,((b,c),d),(#))
时间: 2023-08-18 09:09:57 浏览: 43
以下是用C语言编写程序根据输入的字符串形式表示的广义表,创建广义表,然后再输出广义表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXSIZE 100
typedef struct GLNode {
char data;
struct GLNode *sublist;
struct GLNode *next;
} GLNode, *GList;
// 创建广义表
GList createGList(char *s, int *pos) {
GList L = NULL;
int len = strlen(s);
while (*pos < len && s[*pos] != ')') {
char c = s[(*pos)++];
if (c == '(') {
GList sublist = createGList(s, pos);
if (L == NULL) {
L = (GList) malloc(sizeof(GLNode));
L->data = '\0';
L->sublist = sublist;
L->next = NULL;
} else {
GLNode *p = L;
while (p->next) {
p = p->next;
}
GLNode *node = (GLNode *) malloc(sizeof(GLNode));
node->data = '\0';
node->sublist = sublist;
node->next = NULL;
p->next = node;
}
} else if (c == ',') {
continue;
} else if (c == '#') {
if (L == NULL) {
L = (GList) malloc(sizeof(GLNode));
L->data = '#';
L->sublist = NULL;
L->next = NULL;
} else {
GLNode *p = L;
while (p->next) {
p = p->next;
}
GLNode *node = (GLNode *) malloc(sizeof(GLNode));
node->data = '#';
node->sublist = NULL;
node->next = NULL;
p->next = node;
}
} else {
if (L == NULL) {
L = (GList) malloc(sizeof(GLNode));
L->data = c;
L->sublist = NULL;
L->next = NULL;
} else {
GLNode *p = L;
while (p->next) {
p = p->next;
}
GLNode *node = (GLNode *) malloc(sizeof(GLNode));
node->data = c;
node->sublist = NULL;
node->next = NULL;
p->next = node;
}
}
}
(*pos)++;
return L;
}
// 输出广义表
void printGList(GList L) {
if (L == NULL) {
return;
}
if (L->data == '\0') {
printf("(");
printGList(L->sublist);
printf(")");
} else if (L->data == '#') {
printf("#");
} else {
printf("%c", L->data);
}
if (L->next) {
printf(",");
printGList(L->next);
}
}
int main() {
char s[MAXSIZE];
printf("请输入广义表的字符串表示:");
scanf("%s", s);
int pos = 0;
GList L = createGList(s, &pos);
printf("广义表为:");
printGList(L);
printf("\n");
return 0;
}
```
示例输入:
```
(a,((b,c),d),(#))
```
示例输出:
```
广义表为:(a,((b,c),d),(#))
```
相关推荐
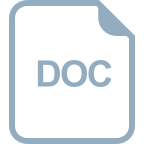
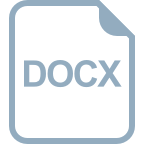
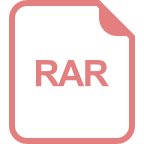
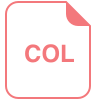
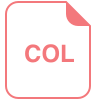
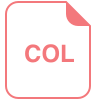
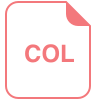
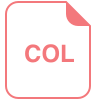









