树结构用C语言写完整广义表形式代码
时间: 2023-06-17 17:01:59 浏览: 56
下面是使用C语言实现广义表的树结构的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义广义表结点
typedef struct GNode {
int tag; // 结点标志,0表示原子,1表示子表
union {
char data; // 存放原子值
struct GNode* hp; // 存放子表头指针
} atom;
struct GNode* tp; // 存放子表尾指针
} GNode;
// 创建结点
GNode* createNode(int tag) {
GNode* node = (GNode*)malloc(sizeof(GNode));
if (node == NULL) {
printf("Memory allocation failed.");
exit(1);
}
node->tag = tag;
node->tp = NULL;
return node;
}
// 创建原子结点
GNode* createAtom(char data) {
GNode* node = createNode(0);
node->atom.data = data;
return node;
}
// 创建子表结点
GNode* createSubList(GNode* hp, GNode* tp) {
GNode* node = createNode(1);
node->atom.hp = hp;
node->tp = tp;
return node;
}
// 解析字符串,构建广义表
GNode* buildGList(char* str, int len, int* index) {
GNode* node = NULL;
if (index == NULL) {
int i = 0;
index = &i;
}
while (*index < len) {
char c = str[*index];
if (c == '(') {
(*index)++;
GNode* hp = buildGList(str, len, index);
GNode* tp = NULL;
if (str[*index] == ',') {
(*index)++;
tp = buildGList(str, len, index);
}
if (str[*index] != ')') {
printf("Invalid input.");
exit(1);
}
(*index)++;
node = createSubList(hp, tp);
break;
}
else if (c == ')') {
break;
}
else if (c == ',') {
printf("Invalid input.");
exit(1);
}
else {
node = createAtom(c);
(*index)++;
break;
}
}
return node;
}
// 打印广义表
void printGList(GNode* node) {
if (node == NULL) {
return;
}
if (node->tag == 0) {
printf("%c", node->atom.data);
}
else {
printf("(");
printGList(node->atom.hp);
if (node->tp != NULL) {
printf(",");
printGList(node->tp);
}
printf(")");
}
}
// 销毁广义表
void destroyGList(GNode* node) {
if (node == NULL) {
return;
}
if (node->tag == 1) {
destroyGList(node->atom.hp);
destroyGList(node->tp);
}
free(node);
}
int main() {
char str[100];
printf("Input a generalized list: ");
scanf("%s", str);
int len = strlen(str);
int index = 0;
GNode* root = buildGList(str, len, &index);
printf("The generalized list is: ");
printGList(root);
printf("\n");
destroyGList(root);
return 0;
}
```
这个代码实现了广义表的创建、打印和销毁功能,使用前需要输入一个广义表的字符串表示。例如,输入字符串`(a,(b,c),d)`,就可以得到如下输出:
```
The generalized list is: (a,(b,c),d)
```
相关推荐
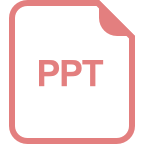
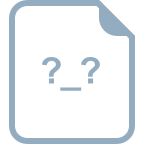














