std::string 查找换行符
时间: 2023-12-13 11:34:17 浏览: 452
可以使用`std::string`的`find`函数来查找换行符。具体实现如下:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello\nworld\n";
size_t pos = str.find('\n'); // 查找第一个换行符的位置
if (pos != std::string::npos) { // 如果找到了
std::cout << "Found newline at position " << pos << std::endl;
} else { // 如果没找到
std::cout << "No newline found" << std::endl;
}
return 0;
}
```
上述代码中,我们首先定义了一个`std::string`类型的变量`str`,并初始化为`"Hello\nworld\n"`。然后,我们使用`find`函数查找第一个换行符的位置,并将结果保存在`pos`变量中。如果找到了,就输出换行符的位置;否则,输出提示信息。
相关问题
std::string识别出xml换行
如果你想在`std::string`中识别出XML换行符,即`\n`和`\r`,你可以使用`std::string::find()`函数来查找并提取每一行的内容。下面是一个示例代码,可以将文本解析为XML格式并提取每一行的内容:
```c++
std::string xml = "<root>\n\t<tag1>\n\t\tvalue1\n\t</tag1>\n\t<tag2>\n\t\tvalue2\n\t</tag2>\n</root>";
std::string line;
std::size_t start_pos = 0;
std::size_t end_pos = 0;
while ((end_pos = xml.find("\n", start_pos)) != std::string::npos) {
line = xml.substr(start_pos, end_pos - start_pos);
// do something with the line, e.g. print it
std::cout << line << std::endl;
start_pos = end_pos + 1;
}
line = xml.substr(start_pos);
// print the last line
std::cout << line << std::endl;
```
这段代码会输出以下内容:
```
<root>
<tag1>
value1
</tag1>
<tag2>
value2
</tag2>
</root>
```
你可以根据自己的需要修改代码,比如将每一行的内容存储到一个数组或容器中,或者对每一行进行进一步处理。
c++ string 删除换行符
在 C++ 中,如果你想要从 `std::string` 中删除换行符(通常是 `\n`),你可以使用标准库提供的函数。这里有几个选项:
1. 使用 `erase()` 和 `find()` 函数:
```cpp
#include <string>
#include <algorithm>
std::string str = "Hello\nWorld!";
str.erase(str.find("\n"), 1); // 找到 '\n' 的位置并删除它
```
这将移除字符串中的第一个换行符。
2. 使用 `replace()` 函数替换换行符为其他字符:
```cpp
str.replace(str.find("\n"), 1, ""); // 将换行符替换成空字符
```
这会直接覆盖换行符的位置。
3. 如果你想删除所有的换行符,可以先查找所有换行符的位置,然后逐个删除:
```cpp
size_t pos = 0;
while ((pos = str.find("\n", pos)) != std::string::npos) {
str.erase(pos, 1);
pos++; // 更新查找起始位置,跳过刚刚删除的字符
}
```
记得包含 `<algorithm>` 或 `<cctype>` 标准库头文件,因为它们包含了 `find()` 和 `isspace()`(判断是否为空格或制表符等)函数。
阅读全文
相关推荐
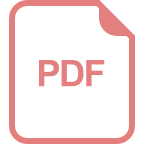
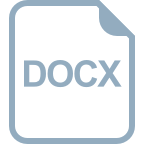
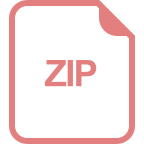













