argument of type 'numpy.float64' is not iterable
时间: 2024-02-01 08:04:37 浏览: 153
这个错误通常出现在你尝试将一个单独的标量(scalar)值作为迭代器来使用时。在 NumPy 中,一些函数可能会返回单独的标量值,而不是数组或其他可迭代对象,这时如果你尝试将其作为迭代器来使用,就会出现这个错误。
例如,下面的代码将会出现这个错误:
```python
import numpy as np
# 创建一个标量(scalar)值
x = np.float64(1.0)
# 尝试将标量值作为迭代器来使用
for xi in x:
print(xi)
```
运行代码会出现以下错误信息:
```
TypeError: argument of type 'numpy.float64' is not iterable
```
如果你需要将标量值转换为可迭代对象,你可以使用 `numpy.array()` 函数来创建一个只包含该标量值的 NumPy 数组,例如:
```python
import numpy as np
# 创建一个标量(scalar)值
x = np.float64(1.0)
# 将标量值转换为包含单个元素的NumPy数组
x_arr = np.array([x])
# 现在可以将其作为迭代器来使用
for xi in x_arr:
print(xi)
```
输出结果为:
```
1.0
```
这里,我们首先使用 `np.array()` 函数将标量值转换为一个只包含该值的数组,然后使用该数组作为迭代器。
相关问题
TypeError: argument of type 'numpy.float64' is not iterable
This error occurs when you try to iterate over a numpy float64 object, which is not iterable.
For example, the following code will raise this error:
```python
import numpy as np
x = np.float64(3.14)
for i in x:
print(i)
```
To fix this error, you need to convert the numpy float64 object to an iterable object such as a list or an array.
For example:
```python
import numpy as np
x = np.float64(3.14)
lst = [x] # Convert x to a list
for i in lst:
print(i)
```
Alternatively, you may want to check your code and make sure that you are not accidentally trying to iterate over a numpy float64 object when you meant to iterate over a list or an array.
argument of type 'numpy.int64' is not iterable
这个错误信息表明你在Python代码中尝试迭代一个`numpy.int64`类型的对象,但是`numpy.int64`类型的对象并不是一个可迭代对象。在Python中,可迭代对象通常是指像列表、元组、字典、集合或者迭代器这样的对象,它们可以返回它们的成员元素。
举个例子,如果你有一个`numpy.int64`类型的变量,并尝试用`for`循环来迭代它,你会遇到这个错误:
```python
import numpy as np
x = np.int64(10)
for i in x:
print(i)
```
上面的代码会导致错误,因为`x`是一个整数,不是一个可迭代对象。
解决方法是检查代码中是否错误地将一个整数用在了需要可迭代对象的地方。如果你确实需要迭代某个范围内的数字,应该使用`range()`函数来创建一个可迭代的序列。例如:
```python
import numpy as np
x = np.int64(10)
for i in range(x): # range(10) 会生成一个从0到9的整数序列
print(i)
```
在这个例子中,`range(x)`生成了一个从0到`x-1`的整数序列,这些整数是可以迭代的。
阅读全文
相关推荐
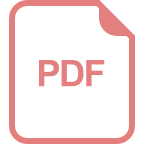
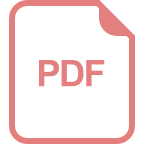













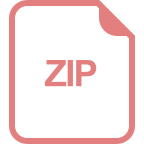